以类的形式使用pymysql写学生管理系统
时间: 2024-09-23 14:16:37 浏览: 47
在Python中使用pymysql库构建一个简单的学生管理系统,通常会涉及设计类来表示学生、课程等实体,并通过类的方法来管理数据库操作。这里是一个基本的概念示例:
首先,安装pymysql库,如果尚未安装,可以使用`pip install pymysql`命令。
```python
import pymysql
# 创建连接数据库的类
class DatabaseConnection:
def __init__(self, host, user, password, db):
self.conn = pymysql.connect(host=host, user=user, password=password, database=db)
self.cursor = self.conn.cursor()
def execute_query(self, sql):
try:
self.cursor.execute(sql)
self.conn.commit()
except Exception as e:
print(f"Error executing query: {e}")
self.conn.rollback()
def close_connection(self):
self.cursor.close()
self.conn.close()
# 定义学生类
class Student:
def __init__(self, id, name, grade):
self.id = id
self.name = name
self.grade = grade
# 添加学生到数据库的方法
@classmethod
def add_student(cls, connection, student_data):
insert_sql = "INSERT INTO students (id, name, grade) VALUES (%s, %s, %s)"
connection.execute_query(insert_sql, student_data)
# 获取所有学生的列表方法
@classmethod
def get_all_students(cls, connection):
select_sql = "SELECT * FROM students"
connection.execute_query(select_sql)
return [Student(*row) for row in connection.cursor.fetchall()]
# 示例用法
if __name__ == "__main__":
db_conn = DatabaseConnection("localhost", "your_username", "your_password", "student_management")
# 添加新学生
new_student = Student(1, "张三", 9)
Student.add_student(db_conn, (new_student.id, new_student.name, new_student.grade))
# 获取并打印所有学生
all_students = Student.get_all_students(db_conn)
for student in all_students:
print(student.name)
db_conn.close_connection()
```
在这个例子中,我们创建了两个类:`DatabaseConnection`用于处理数据库操作,而`Student`则包含学生的基本信息以及与数据库交互的方法。在实际项目中,可以根据需求添加更多的功能,如删除、更新学生信息等。
阅读全文
相关推荐
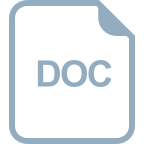
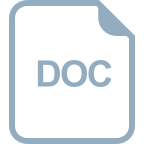
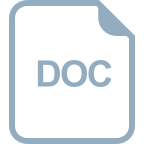
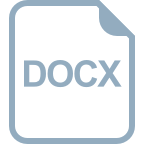


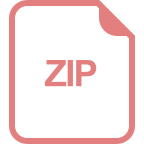
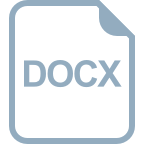
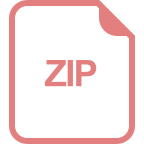
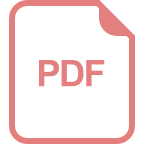
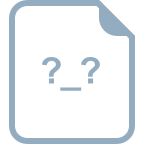
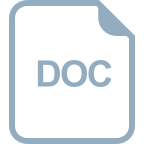
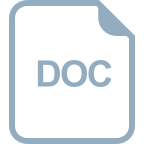
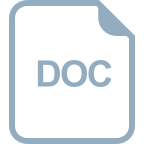
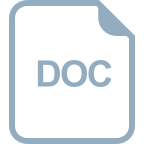



