C# 根据输入int值返回大写字母表对应的字母,从A开始
时间: 2024-09-26 10:15:56 浏览: 33
在C#中,你可以创建一个函数,该函数接受一个整数作为输入,然后使用取模运算(%)来确定在英文字母表中的位置,并根据这个位置返回相应的大写字母。这里是一个简单的示例:
```csharp
using System;
public static string Get对应字母(int number)
{
if (number < 0 || number > 25) // 检查是否在字母表范围内
return "输入无效";
// A 的 ASCII 值是 65,所以我们可以用 number 对 26 取模得到大写字母表的位置
char letter = (char)(('A' + number - 1) % 26 + 'A');
return(letter.ToString());
}
```
这个函数假设输入的数字在0到25之间(因为大写字母表只有26个字母)。对于超出范围的输入,它会返回"输入无效"。
使用例子:
```csharp
Console.WriteLine(Get对应字母(1)); // 输出:A
Console.WriteLine(Get对应字母(27)); // 输出:B
Console.WriteLine(Get对应字母(-1)); // 输出:输入无效
```
相关问题
C#输入一个小字母,输出一个相对应的大写字母
可以使用 C# 中的 Char.ToUpper() 方法将小写字母转换为大写字母。以下是一个简单的示例代码:
```
char input = 'a';
char output = Char.ToUpper(input);
Console.WriteLine(output);
```
输出结果为:A
如果要将用户输入的小写字母转换为大写字母,可以使用 Console.ReadLine() 方法获取用户输入的字符串,然后使用 Char.ToUpper() 方法将第一个字符转换为大写字母。以下是一个示例代码:
```
Console.WriteLine("请输入一个小写字母:");
string input = Console.ReadLine();
char firstChar = input[0];
char output = Char.ToUpper(firstChar);
Console.WriteLine("转换后的大写字母为:" + output);
```
c#验证输入的值只能以字母开头
在 C# 中,你可以使用正则表达式 (Regular Expression) 和属性验证 (Data Validation) 来检查用户输入是否只允许字母开头。以下是一个简单的示例:
```csharp
using System.ComponentModel.DataAnnotations;
public class MyModel
{
[RegularExpression(@"^[a-zA-Z]")] // 正则表达式,匹配以字母开头的内容
public string InputField { get; set; }
}
```
在这个例子中,`InputField` 属性被标记为 `[RegularExpression]`,它会检查输入是否符合给定的正则模式 `^[a-zA-Z]`,该模式仅允许从 A 到 Z 或 a 到 z 的任意单个字母。
如果你想在控制器层进行更复杂的验证,可以利用 `Validator` 类,例如:
```csharp
if (!ModelState.IsValid)
{
// 输入字段验证失败,处理错误...
}
```
阅读全文
相关推荐
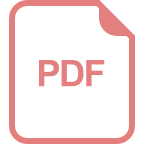
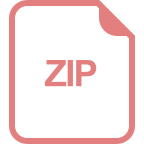
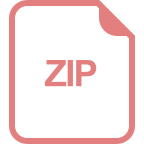













