cpp实现创造一个整数并限制在大于等于0范围,任何将其变为负数的操作都会失效
时间: 2024-10-25 14:17:07 浏览: 18
在C++中,你可以创建一个类来实现这个功能,其中包含一个私有成员变量表示实际数值,并重载运算符使其只允许非负操作。这里有一个简单的示例:
```cpp
class PositiveInteger {
private:
int value;
public:
PositiveInteger(int num = 0) : value(std::max(0, num)) {}
// 重载加法运算符,保证结果是非负
PositiveInteger operator+(PositiveInteger other) const {
return PositiveInteger(value + other.value);
}
// 其他必要的运算符重载,如减法、乘法等,都需要保证结果非负
// 保护性构造,不允许直接赋值为负数
void operator=(int num) {
if (num < 0) {
throw std::invalid_argument("Cannot assign a negative value to a PositiveInteger.");
}
value = std::max(0, num);
}
// 返回当前值
int get_value() const { return value; }
};
// 示例用法
int main() {
PositiveInteger pi(10); // 创建一个初始值为10的正整数
try {
pi = -5; // 这会抛出异常,因为尝试将负数赋给PositiveInteger
} catch (const std::invalid_argument& e) {
std::cout << "Error: " << e.what() << '\n';
}
PositiveInteger result = pi + 5; // 正确操作,返回一个新的PositiveInteger
std::cout << "Result: " << result.get_value() << '\n'; // 输出:15
return 0;
}
```
阅读全文
相关推荐
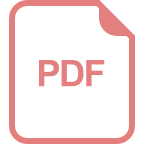
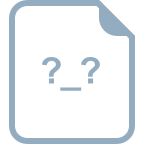
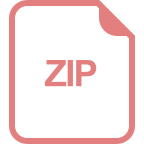
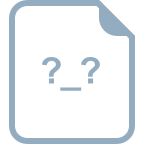
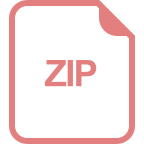