springcloud aop
时间: 2023-09-01 21:08:03 浏览: 75
Spring Cloud AOP(面向切面编程)是 Spring Cloud 框架中的一个核心组件,它提供了一种基于切面的编程方式来实现横切关注点的解耦和复用。
AOP 是一种编程范式,它允许开发人员通过将横切关注点从业务逻辑中剥离出来,以模块化的方式来处理通用的横切关注点,例如日志记录、安全性、事务管理等。在 Spring Cloud 中使用 AOP,您可以通过定义切面和连接点来实现这些功能。
在 Spring Cloud 中使用 AOP,您需要引入相关的依赖并配置切面和切点。通过在切面中定义通知(advice),您可以指定在程序执行到特定连接点时要执行的代码。常见的通知类型包括前置通知(Before)、后置通知(After)、返回通知(AfterReturning)和异常通知(AfterThrowing)。
以下是一个使用 Spring Cloud AOP 的示例:
```java
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Before method: " + joinPoint.getSignature().getName());
}
@After("execution(* com.example.service.*.*(..))")
public void logAfter(JoinPoint joinPoint) {
System.out.println("After method: " + joinPoint.getSignature().getName());
}
@AfterReturning(pointcut = "execution(* com.example.service.*.*(..))", returning = "result")
public void logAfterReturning(JoinPoint joinPoint, Object result) {
System.out.println("After returning method: " + joinPoint.getSignature().getName());
}
@AfterThrowing(pointcut = "execution(* com.example.service.*.*(..))", throwing = "exception")
public void logAfterThrowing(JoinPoint joinPoint, Exception exception) {
System.out.println("After throwing method: " + joinPoint.getSignature().getName());
}
}
```
上述示例中,我们定义了一个名为 `LoggingAspect` 的切面,并在切面中定义了四个通知方法。通过 `@Before`、`@After`、`@AfterReturning` 和 `@AfterThrowing` 注解,我们分别实现了前置通知、后置通知、返回通知和异常通知。这些通知方法会在连接点(这里是 `com.example.service` 包下的所有方法)执行前、后、返回和抛出异常时被触发。
需要注意的是,要使 AOP 生效,您还需要在 Spring Cloud 应用程序的配置文件中启用 AOP,例如,在 `application.properties` 中添加以下配置:
```
spring.aop.auto=true
```
这样,Spring Cloud 应用程序中的相关切面和连接点就会被自动扫描并应用。希望这个简单的示例能帮助您理解 Spring Cloud AOP 的基本用法。如果您有更多问题,请随时提问!
相关推荐
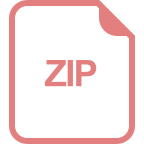
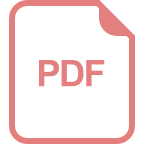
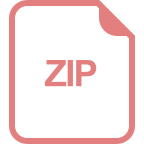














