C++语言,创建一个string数组,用于获取5个随机输入字符串,输出5个字符串中包含数字最多的字符串与长度最长的字符串(不考虑出现并列第一的情况)c++
时间: 2024-09-06 13:04:53 浏览: 18
在C++中,你可以使用`<iostream>`、`<vector>`、`<algorithm>`以及`<cstdlib>`库来实现这个功能。首先,你需要从用户那里获取五个随机生成的输入字符串,然后遍历数组找出包含数字最多和长度最长的字符串。下面是一个简单的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
#include <cstdlib>
#include <ctime>
std::string getRandomString(int length) {
std::srand(std::time(0)); // 设置随机数种子
std::string result;
for (int i = 0; i < length; ++i) {
result += 'A' + static_cast<char>(std::rand() % 26); // 生成大写字母
}
return result;
}
// 函数用于比较两个字符串的特性(包含的数字数量和长度)
struct CompareStrings {
bool operator()(const std::string& a, const std::string& b) const {
int numDigitsA = count(a.begin(), a.end(), '0') + count(a.begin(), a.end(), '1') + count(a.begin(), a.end(), '2') + ...; // 计算a中的数字数量
int numDigitsB = count(b.begin(), b.end(), '0') + count(b.begin(), b.end(), '1') + count(b.begin(), b.end(), '2') + ...;
if (numDigitsA > numDigitsB) {
return true;
} else if (numDigitsA == numDigitsB && a.length() > b.length()) {
return true;
}
return false;
}
};
int main() {
std::vector<std::string> strings(5);
for (int i = 0; i < 5; ++i) {
strings[i] = getRandomString(rand() % 10 + 5); // 随机生成长度在5到14之间的字符串
}
// 使用lambda表达式和sort函数找到包含数字最多的字符串
auto maxDigitsStr = *max_element(strings.begin(), strings.end(), CompareStrings());
// 再次使用lambda表达式和sort函数找到长度最长的字符串(这里假设已知maxDigitsStr不是最长的,所以会排除它)
auto longestLengthStr = *(std::find_if_not(strings.begin(), strings.end(), [maxDigitsStr](const auto& s) { return s == maxDigitsStr; }));
std::cout << "包含数字最多的字符串是: " << maxDigitsStr << std::endl;
std::cout << "长度最长的字符串是: " << longestLengthStr << std::endl;
return 0;
}
```
相关推荐
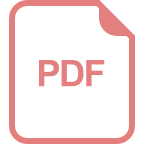
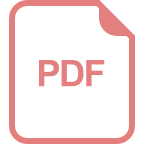
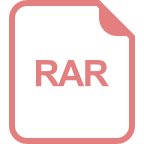
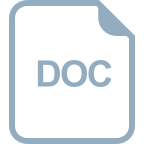
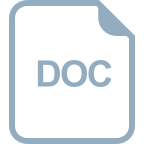
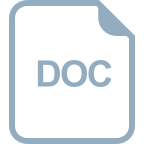
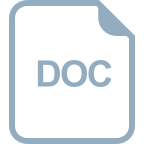
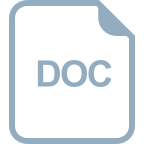
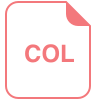
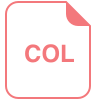
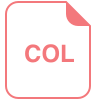
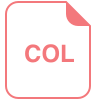
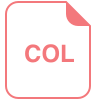





