qt设置透明的光标样式后,需要移动一下鼠标才能隐藏
时间: 2024-04-16 16:26:55 浏览: 11
你可以尝试在设置透明光标样式后调`QCursor::setPos()`方法来移动一下鼠标位置,以便隐藏光标。这个方法可以将鼠标光标移动到指定的屏幕坐标位置。你可以将位置设置在当前鼠标位置的附近,这样就能够实现隐藏光标的效果。
下面是一个示例代码片段:
```cpp
// 设置透明光标样式
QPixmap cursorPixmap(16, 16);
cursorPixmap.fill(Qt::transparent);
QCursor transparentCursor(cursorPixmap);
// 设置光标样式
QWidget::setCursor(transparentCursor);
// 移动鼠标以隐藏光标
QPoint currentPos = QCursor::pos();
QCursor::setPos(currentPos + QPoint(1, 1));
```
你可以将这段代码放在设置透明光标样式的地方,这样在设置完样式后,鼠标会稍微移动一下,从而达到隐藏光标的效果。
相关问题
C++Qt实现给QcustomPlot对象添加光标,使得光标随着鼠标移动
可以使用QMouseEvent来监听鼠标移动事件,然后根据鼠标位置来调整光标的位置。具体实现步骤如下:
1. 在QCustomPlot对象上添加一个QCPItemStraightLine对象作为光标,并设置好光标的样式和位置。
```cpp
QCPItemStraightLine *cursor = new QCPItemStraightLine(customPlot);
cursor->setPen(QPen(Qt::red));
cursor->point1->setCoords(0, 0);
cursor->point2->setCoords(0, 1);
```
2. 在QCustomPlot对象上监听鼠标移动事件,获取鼠标位置,然后根据鼠标位置来调整光标的位置。
```cpp
customPlot->setMouseTracking(true); // 开启鼠标移动事件监听
connect(customPlot, &QCustomPlot::mouseMove, [cursor](QMouseEvent *event){
double x = cursor->point1->coords().x(); // 获取当前光标的x坐标
double y = event->pos().y(); // 获取鼠标的y坐标
cursor->point1->setCoords(x, y);
cursor->point2->setCoords(x, y);
cursor->setVisible(true); // 显示光标
});
```
这样,就可以实现给QCustomPlot对象添加光标,并使光标随着鼠标移动的效果了。
C++Qt不用UI的形式给QCustomPlot编写的图形添加光标,使得光标能够跟随鼠标移动
可以通过在QCustomPlot的replot()函数中添加一个事件过滤器,实时监听鼠标位置并更新光标的位置实现光标跟随鼠标移动的效果。具体实现步骤如下:
1. 在构造函数中初始化光标对象,设置光标样式和位置。
```c++
MyCustomPlot::MyCustomPlot(QWidget *parent)
: QCustomPlot(parent)
{
// 初始化光标对象
mCursorLine = new QCPItemStraightLine(this);
mCursorLine->setPen(QPen(Qt::red));
mCursorLine->setLayer("overlay"); // 设置光标在顶层
// 将光标对象添加到绘图区域
addItem(mCursorLine);
// 设置光标位置
mCursorLine->point1->setCoords(0, yAxis->range().lower);
mCursorLine->point2->setCoords(0, yAxis->range().upper);
}
```
2. 在QCustomPlot的replot()函数中添加事件过滤器,实时监听鼠标位置并更新光标的位置。
```c++
void MyCustomPlot::replot(QCustomPlot::RefreshPriority refreshPriority)
{
// 添加事件过滤器,实时监听鼠标位置并更新光标的位置
installEventFilter(this);
// 调用父类的replot()函数
QCustomPlot::replot(refreshPriority);
}
```
3. 在事件过滤器中实现鼠标位置的监听和光标位置的更新。
```c++
bool MyCustomPlot::eventFilter(QObject *obj, QEvent *event)
{
if (event->type() == QEvent::MouseMove)
{
// 获取鼠标在绘图区域中的位置
QMouseEvent *mouseEvent = static_cast<QMouseEvent*>(event);
QPointF pos = mouseEvent->posF();
double x = xAxis->pixelToCoord(pos.x());
// 更新光标位置
mCursorLine->point1->setCoords(x, yAxis->range().lower);
mCursorLine->point2->setCoords(x, yAxis->range().upper);
// 重新绘制绘图区域
replot();
return true;
}
// 将事件传递给父类处理
return QCustomPlot::eventFilter(obj, event);
}
```
通过上述步骤,就可以实现不用UI的形式给QCustomPlot编写的图形添加光标,使得光标能够跟随鼠标移动了。
相关推荐
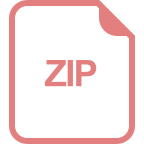
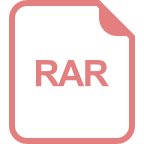
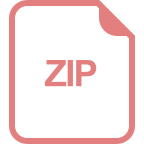












