请写出使用RestTemplate通过服务发现,基于注册中心完成远程调用的流程
时间: 2024-04-12 21:31:46 浏览: 10
使用RestTemplate通过服务发现,基于注册中心完成远程调用的流程可以分为以下几个步骤:
1. 在应用程序中配置注册中心信息:在应用程序的配置文件中,添加注册中心的相关配置,包括注册中心的地址、端口等信息。
2. 创建RestTemplate实例:在应用程序中创建一个RestTemplate的实例,用于发送HTTP请求。
3. 通过服务发现获取服务实例:使用服务发现功能,根据服务名称从注册中心获取可用的服务实例。服务发现可以使用Eureka、Consul等开源组件,或者使用Spring Cloud的服务发现功能。
4. 使用RestTemplate发送远程调用请求:通过RestTemplate发送HTTP请求到获取的服务实例。可以使用RestTemplate的各种方法,如getForObject、postForObject等,根据具体需求选择合适的方法。
下面是一个简单的示例代码,演示了如何使用RestTemplate通过服务发现完成远程调用:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cloud.client.ServiceInstance;
import org.springframework.cloud.client.discovery.DiscoveryClient;
import org.springframework.stereotype.Component;
import org.springframework.web.client.RestTemplate;
@Component
public class RemoteServiceInvoker {
@Autowired
private DiscoveryClient discoveryClient;
private RestTemplate restTemplate = new RestTemplate();
public void invokeRemoteService(String serviceName, String endpoint) {
// 通过服务发现获取服务实例
List<ServiceInstance> instances = discoveryClient.getInstances(serviceName);
if (instances.isEmpty()) {
throw new RuntimeException("No instances available for service: " + serviceName);
}
// 选择一个服务实例
ServiceInstance instance = instances.get(0);
String url = instance.getUri().toString() + endpoint;
// 使用RestTemplate发送远程调用请求
String response = restTemplate.getForObject(url, String.class);
System.out.println("Response: " + response);
}
}
```
在上述示例中,通过@Autowired注解注入了DiscoveryClient,用于获取服务实例。然后通过getInstances方法获取指定服务名称的实例列表,选择其中一个实例,并获取其URI。最后使用RestTemplate发送HTTP请求,调用远程服务的接口。
请注意,上述示例只是一个简单的示例,实际应用中还需要考虑错误处理、负载均衡等问题。具体的实现方式可能会因为使用的注册中心组件不同而有所差异。
相关推荐
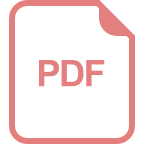
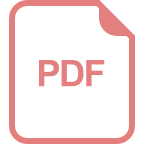



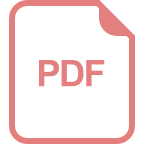
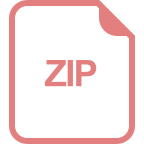
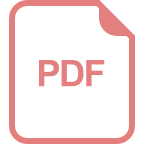
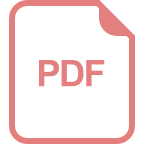
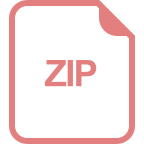
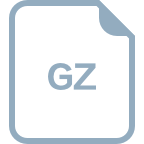
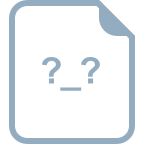
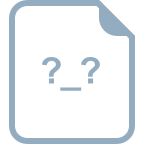