用c语言在计算机上完成线性表链式存储结构创建,实现插入删除
时间: 2024-11-24 16:40:37 浏览: 27
在C语言中,链式存储结构常用于线性表的表示,因为它不需要连续的内存空间。下面我会为你简单地展示如何创建链表以及插入和删除节点的基本步骤。
首先,我们需要定义一个链表节点的数据结构:
```c
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
```
接下来,我们创建链表的函数可以这样设计:
```c
// 创建空链表
Node* createList() {
return NULL; // 初始化为空
}
// 插入节点到链表的头部
void insertAtBeginning(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 分配内存
newNode->data = value;
newNode->next = *head; // 新节点指向头节点
*head = newNode; // 更新头节点指向新节点
}
// 删除特定值的节点
void deleteNode(Node** head, int value) {
if (*head == NULL) { // 如果链表为空
return;
}
if ((*head)->data == value) { // 如果头节点就是要删除的
Node* temp = *head;
*head = (*head)->next; // 更新头节点
free(temp); // 释放原头节点内存
return;
}
Node* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) { // 找到了要删除的节点
Node* temp = current->next;
current->next = current->next->next; // 跳过要删除的节点
free(temp); // 释放被删除节点的内存
}
}
```
阅读全文
相关推荐
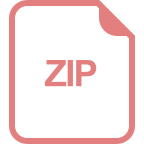
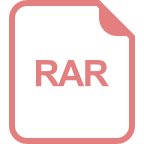
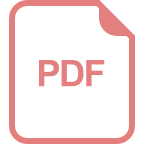
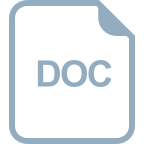


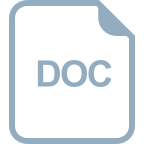




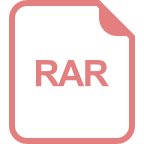
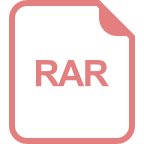
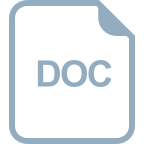
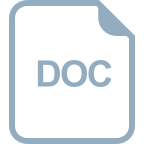



