python写购物车管理
时间: 2024-12-19 12:19:42 浏览: 6
在Python中编写一个简单的购物车管理系统,通常会包括以下几个关键部分:
1. **商品类**(Product): 这是一个基本的数据结构,包含商品名称、价格等属性。
```python
class Product:
def __init__(self, name, price):
self.name = name
self.price = price
```
2. **购物车类**(ShoppingCart): 负责存储商品,包含添加商品、删除商品、计算总价等功能。
```python
class ShoppingCart:
def __init__(self):
self.items = {}
def add_item(self, product_name, quantity=1):
if product_name in self.items:
self.items[product_name] += quantity
else:
self.items[product_name] = quantity
def remove_item(self, product_name, quantity=None):
if product_name in self.items and (quantity is None or quantity <= self.items[product_name]):
self.items[product_name] -= quantity
if self.items[product_name] == 0:
del self.items[product_name]
def total_cost(self):
return sum(item["price"] * item["quantity"] for item in self.items.values())
```
3. **主程序示例**:
```python
# 使用例子
cart = ShoppingCart()
cart.add_item("苹果", 5)
cart.add_item("香蕉", 2)
print(f"总价格: {cart.total_cost()}元")
cart.remove_item("苹果", 3)
print(f"修改后,苹果剩余{cart.items['苹果']}, 总价格: {cart.total_cost()}元")
```
阅读全文
相关推荐



















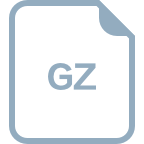