Serial port not selected
时间: 2024-08-15 15:09:38 浏览: 60
串口未选择通常是指在计算机系统中,当你试图通过串行通信(如RS-232、USB到串口转换器等)连接设备时,系统的串口设置没有被正确配置或选定为活跃端口。这可能是由于以下几个原因:
1. **硬件连接**:检查串口电缆是否已正确插入,并确认设备是否已经连上串口。
2. **驱动程序**:确保串口相关的驱动程序已安装并更新至最新版本,尤其是对于旧电脑或新设备可能需要特别注意。
3. **系统设置**:在操作系统控制面板或终端里,确认"设备管理器"中的"端口(COM和LPT)"部分,串口应被识别并勾选为"启用"状态。
4. **软件配置**:在需要使用的应用程序中,查找串口配置选项,可能需要手动指定正确的端口号。
5. **权限问题**:某些情况下,用户账户可能没有权限访问特定的串口,需要管理员权限去修改设置。
相关问题
simulink serial send
To send data through a serial port using Simulink, you can use the "Serial Send" block from the Simulink Support Package for Arduino Hardware.
Here are the steps to send data through a serial port using Simulink:
1. Open Simulink and create a new model.
2. From the Simulink Library Browser, navigate to the Simulink Support Package for Arduino Hardware.
3. Drag and drop the "Serial Send" block into your Simulink model.
4. Double-click the "Serial Send" block to open its Block Parameters dialog box.
5. In the "Port" field, select the serial port that you want to use for sending data. If you are not sure which port to use, you can check your computer's Device Manager or System Information utility.
6. In the "Data type" field, select the data type of the data you want to send. For example, if you want to send a single floating-point value, select "single".
7. In the "Sample time" field, specify the rate at which you want to send data. For example, if you want to send data every 1 second, enter "1" in the field.
8. In the "Format string" field, enter the format string that corresponds to the data type you selected in step 6. For example, if you selected "single", enter "%f" in the field.
9. In the "Data" field, enter the data that you want to send. This can be a scalar value, a vector, or a matrix.
10. Save your Simulink model and run the simulation.
Your data should now be sent through the specified serial port at the specified sample time.
Web Serial API 读取一行数据
### 回答1:
Web Serial API 可以通过 `readLine()` 方法读取一行数据。这个方法会一直阻塞,直到读取到一个完整的行(以换行符结尾)或者超时。以下是一个简单的示例代码:
```javascript
async function readLine() {
const reader = port.readable.getReader();
let result = '';
while (true) {
const { value, done } = await reader.read();
if (done) {
throw new Error('Read terminated');
}
result += new TextDecoder().decode(value);
if (result.endsWith('\n')) {
return result.trim();
}
}
}
const port = await navigator.serial.requestPort();
await port.open({ baudRate: 9600 });
await port.readLine(); // 读取一行数据
```
注意:上述代码中的 `port` 对象是通过 `navigator.serial.requestPort()` 方法获取的,并且需要用户授权才能使用。在实际应用中,还需要根据具体的串口设备配置正确的参数,如波特率、数据位、停止位等。
### 回答2:
Web Serial API 是一种在Web浏览器中允许访问串行设备的API。它允许Web应用程序与连接到计算机的串行设备进行通信。要读取一行数据,我们可以按照以下步骤操作:
1. 首先,我们需要从浏览器中请求用户授权以访问串行设备。此时,浏览器会显示一个权限对话框,询问用户是否允许应用程序访问串行设备。
2. 一旦用户授权,我们可以使用`navigator.serial.requestPort()`方法来请求访问串行端口。该方法返回一个Promise,用于处理串行端口的选择。
3. 当用户选择串行端口后,我们可以使用`port.readable`属性来获取一个`ReadableStream`对象。该对象允许我们从串行设备读取数据。
4. 接下来,我们可以通过调用`reader = stream.getReader()`方法来获取一个`ReadableStreamDefaultReader`对象。该对象用于读取数据流中的字节。
5. 通过调用`reader.read()`方法,我们可以读取一行数据。该方法返回一个Promise,它解析为一个包含已读取字节数和数据的对象。
6. 我们可以使用解析的数据,根据需要进行处理或显示。如果我们希望继续读取下一行数据,可以在处理完当前行数据后,再次调用`reader.read()`方法。
总的来说,使用Web Serial API 读取一行数据需要获取用户授权、选择串行端口、获取`ReadableStream`对象、获取`ReadableStreamDefaultReader`对象,然后通过调用`reader.read()`方法来读取数据。
### 回答3:
Web Serial API 可以通过以下步骤读取一行数据:
首先,我们需要通过 navigator.serial.requestPort() 方法请求用户授权以访问串行端口。这将打开一个对话框,显示可用的串行设备端口列表,并允许用户选择一个端口。
一旦用户选择了一个串行端口,我们可以通过返回的 SerialPort 对象中的 .readable 属性来检查该端口是否可读。如果可读,则我们可以通过新建一个 TextDecoder 对象来处理读取数据时的字符解码操作。
接下来,我们可以通过 SerialPort 对象的 .readLine() 方法读取一行数据。该方法会一直阻塞,直到读取到一行完整的数据或遇到读取错误。
读取成功后,我们可以将读取到的数据通过回调函数或其他方式进行处理。注意,读取的数据并不包含行尾符。
最后,我们可以通过调用 SerialPort 对象的 .close() 方法关闭串行端口。
以下是一个简单的示例代码:
```javascript
async function readLineFromSerial() {
try {
const port = await navigator.serial.requestPort();
await port.open({ baudRate: 115200 });
if (!port.readable) {
console.error('The selected port is not readable!');
return;
}
const decoder = new TextDecoder('utf-8');
while (true) {
const reader = port.readable.getReader();
const { value, done } = await reader.read();
if (done) {
console.log('Connection closed.');
break;
}
const line = decoder.decode(value);
console.log('Received:', line);
}
await port.close();
} catch (error) {
console.error('Error:', error);
}
}
readLineFromSerial();
```
上述代码中的例子使用 Web Serial API 的方式打开一个串行端口,并在一个无限循环中读取一行数据,然后将其进行解码和输出。当读取完成或发生错误时,关闭端口。
阅读全文
相关推荐
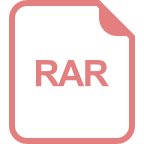
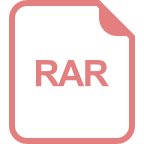
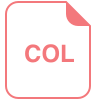

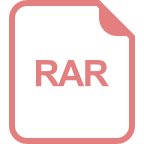
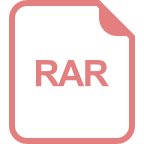
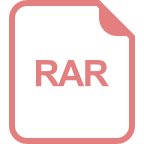