要实现MyString对象的相加,分别用成员函数和友元函数实现+运算符重载,写出重载函数原型。
时间: 2024-10-24 17:13:26 浏览: 26
为了在C++中实现`MyString`对象的自加操作,即两个字符串相加,你可以通过两种方式来重载`+`运算符:成员函数和友元函数。
**1. 成员函数实现**:
```cpp
class MyString {
public:
// 成员函数实现+运算符重载
MyString operator+(const MyString& other) const; // 返回一个新的MyString对象,包含当前对象与other的连接
private:
// 其他成员变量和构造/析构等
};
```
这里的原型声明了`operator+`为一个常量引用类型的`MyString`返回值,它接受另一个`MyString`对象作为参数。
**2. 友元函数实现**:
```cpp
class MyString;
// 定义友元函数
class StringAdder {
public:
static MyString operator+(const MyString& str1, const MyString& str2);
};
// 在MyString类中声明友元关系
class MyString {
friend class StringAdder;
// ...
};
```
在这种情况下,`operator+`不是`MyString`类的一部分,而是外部的`StringAdder`类的一个静态成员函数。
对于这两种情况,你需要提供具体的实现,例如合并两个字符串的内容、分配新的内存空间并复制数据等。
相关问题
用c++实现:设计一个字符串类重载“+=”运算符,使其实现两个字符串的连接并赋值。试分别用成员函数和友元函数实现。
首先,我们定义一个字符串类:
```cpp
#include <cstring>
class MyString {
public:
MyString(const char* str = nullptr);
MyString(const MyString& other);
~MyString();
MyString& operator=(const MyString& other);
const char* c_str() const;
private:
char* m_data;
};
MyString::MyString(const char* str) {
if (str == nullptr) {
m_data = new char[1];
*m_data = '\0';
} else {
m_data = new char[strlen(str) + 1];
strcpy(m_data, str);
}
}
MyString::MyString(const MyString& other) {
m_data = new char[strlen(other.m_data) + 1];
strcpy(m_data, other.m_data);
}
MyString::~MyString() {
delete[] m_data;
}
MyString& MyString::operator=(const MyString& other) {
if (this != &other) {
delete[] m_data;
m_data = new char[strlen(other.m_data) + 1];
strcpy(m_data, other.m_data);
}
return *this;
}
const char* MyString::c_str() const {
return m_data;
}
```
接下来,我们可以用成员函数和友元函数分别实现重载“+=”运算符:
```cpp
// 成员函数实现
MyString& MyString::operator+=(const MyString& other) {
char* temp = new char[strlen(m_data) + strlen(other.m_data) + 1];
strcpy(temp, m_data);
strcat(temp, other.m_data);
delete[] m_data;
m_data = temp;
return *this;
}
// 友元函数实现
MyString operator+(const MyString& str1, const MyString& str2) {
MyString temp;
delete[] temp.m_data;
temp.m_data = new char[strlen(str1.m_data) + strlen(str2.m_data) + 1];
strcpy(temp.m_data, str1.m_data);
strcat(temp.m_data, str2.m_data);
return temp;
}
MyString& MyString::operator+=(const MyString& other) {
*this = *this + other;
return *this;
}
```
使用示例:
```cpp
#include <iostream>
using namespace std;
int main() {
MyString str1("Hello");
MyString str2(" World!");
str1 += str2; // 成员函数实现
cout << str1.c_str() << endl;
MyString str3("Hello");
MyString str4(" C++!");
MyString str5 = str3 + str4; // 友元函数实现
cout << str5.c_str() << endl;
return 0;
}
```
自定义一个MyString类,实现构造,析构,拷贝构造,赋值运算重载、[]运算符重载 +运算符重载 +=运 算符重载 ==运算符重载
自定义一个名为MyString的类,我们可以按照标准步骤实现所需的功能:
1. 构造函数:
```cpp
class MyString {
private:
std::string data;
public:
// 默认构造函数
MyString() : data{} {}
// 拷贝构造函数
MyString(const MyString& other) : data(other.data) {}
// 如果需要,可以提供带初始字符串的构造函数
explicit MyString(const std::string& str) : data(str) {}
};
```
2. 析构函数(destructor):
```cpp
~MyString() {} // 默认析构函数,释放内部资源
```
3. 拷贝构造函数(copy constructor):
```cpp
MyString& operator=(const MyString& other) {
if (this != &other) {
data = other.data;
}
return *this;
}
```
4. 赋值运算符重载(assignment operator):
```cpp
MyString& operator=(std::string&& str) {
data = std::move(str);
return *this;
}
```
5. [] 运算符重载(数组访问):
```cpp
char& operator[](size_t index) {
if (index < data.size()) {
return data[index];
} else {
throw std::out_of_range("Array out of range");
}
}
const char& operator[](size_t index) const {
if (index < data.size()) {
return data[index];
} else {
throw std::out_of_range("Array out of range");
}
}
```
6. + 运算符重载(连接两个字符串):
```cpp
MyString operator+(const MyString& other) const {
MyString result(data);
result.data += other.data;
return result;
}
MyString operator+(const std::string& str) const {
MyString result(data);
result.data += str;
return result;
}
```
7. += 运算符重载(将一个字符串追加到当前字符串):
```cpp
MyString& operator+=(const MyString& other) {
data += other.data;
return *this;
}
MyString& operator+=(const std::string& str) {
data += str;
return *this;
}
```
8. == 运算符重载(比较两个字符串是否相等):
```cpp
bool operator==(const MyString& other) const {
return data == other.data;
}
```
阅读全文
相关推荐
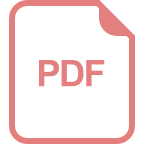
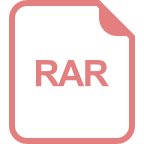
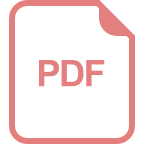













