用java实现ftp
时间: 2024-03-15 20:37:25 浏览: 87
Java可以使用Apache Commons Net库来实现FTP功能。你可以使用FTPClient类来连接FTP服务器并执行各种操作,如上传文件、下载文件、创建文件夹、删除文件等。下面是一个简单的示例代码:
```java
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPReply;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class FTPExample {
public static void main(String[] args) {
String server = "ftp.example.com";
int port = 21;
String username = "username";
String password = "password";
FTPClient ftp = new FTPClient();
try {
// 连接FTP服务器
ftp.connect(server, port);
int reply = ftp.getReplyCode();
if (!FTPReply.isPositiveCompletion(reply)) {
System.out.println("FTP server refused connection.");
return;
}
// 登录
if (!ftp.login(username, password)) {
System.out.println("FTP login failed.");
return;
}
// 设置文件传输模式为二进制
ftp.setFileType(FTPClient.BINARY_FILE_TYPE);
// 上传文件
File localFile = new File("local-file.txt");
FileInputStream inputStream = new FileInputStream(localFile);
ftp.storeFile("remote-file.txt", inputStream);
inputStream.close();
// 下载文件
File downloadFile = new File("download-file.txt");
FileOutputStream outputStream = new FileOutputStream(downloadFile);
ftp.retrieveFile("remote-file.txt", outputStream);
outputStream.close();
// 创建文件夹
ftp.makeDirectory("new-folder");
// 删除文件
ftp.deleteFile("file-to-delete.txt");
// 登出并断开连接
ftp.logout();
ftp.disconnect();
System.out.println("FTP operations completed successfully.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐







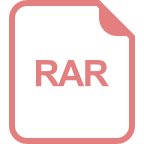

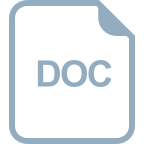



