des加密解密算法,详细的实现,需要完整的代码,用c写
时间: 2024-12-24 15:24:48 浏览: 9
DES (Data Encryption Standard) 是一种对称加密算法,用于保护数据隐私。以下是DES加密和解密的基本流程和简单的C语言实现示例,注意这只是一个基础版本,实际生产环境会更复杂,包含错误处理、填充等步骤:
```c
#include <stdio.h>
#include <string.h>
// DES密码字典长度
#define KEY_LENGTH 8
// DES操作函数,这里仅作说明,真实实现需依赖第三方库如OpenSSL
void DES_encrypt(char* plaintext, char* ciphertext, const char* key);
void DES_decrypt(char* ciphertext, char* plaintext, const char* key);
// 简化的DES加密函数,假设key是64位
void DES_encrypt(char* plaintext, char* ciphertext, const char* key) {
// 这里省略了真正的DES加密步骤,因为这个函数只是演示
// 实际上你需要调用相应的加密API,并输入明文、密钥和输出位置
printf("Encrypting using DES with key: %s\n", key);
// 示例:ciphertext = DES(plaintext, key);
}
// 简化的DES解密函数,同样假设key是64位
void DES_decrypt(char* ciphertext, char* plaintext, const char* key) {
// 解密过程类似,也是假设有现成的解密API
printf("Decrypting using DES with key: %s\n", key);
// 示例:plaintext = DES(ciphertext, key);
}
int main() {
char plaintext[50], ciphertext[50];
const char* key = "your_des_key"; // 请替换为实际的8字节密钥
// 明文
strcpy(plaintext, "Hello, DES!");
// 加密
DES_encrypt(plaintext, ciphertext, key);
// 输出加密后的文本
printf("Encrypted text: %s\n", ciphertext);
// 解密
DES_decrypt(ciphertext, plaintext, key);
// 输出解密后的文本
printf("Decrypted text: %s\n", plaintext);
return 0;
}
```
阅读全文
相关推荐
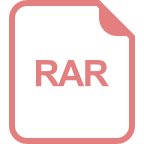
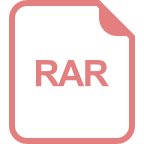
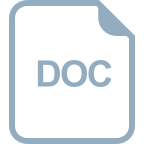
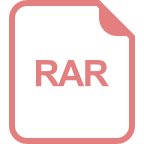
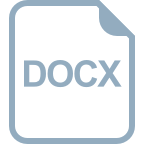
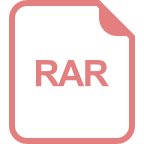
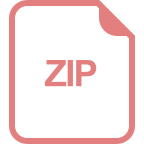

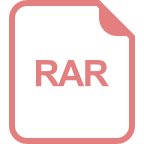
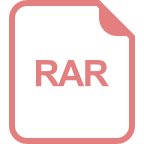
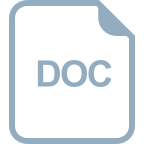
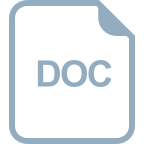
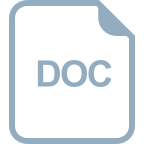