C语言编写代码(1)根据输入顺序表的长度n和各个数据元素值建立一个顺序表,并输出顺序表中各元素值,观察输入的内容与输出的内容是否一致。
时间: 2024-10-09 17:01:22 浏览: 24
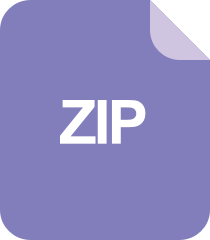
数据结构顺序表 C语言代码实现以及realloc的使用
在C语言中,你可以通过以下几个步骤来实现这个功能:
1. 定义结构体(如果顺序表是自定义的数据类型):
```c
typedef struct {
int data; // 数据域
struct Node* next; // 指向下一个元素的指针
} Node;
```
2. 创建顺序表:
```c
void createList(int n, int elements[]) {
Node* head = NULL; // 初始化头节点为NULL
for (int i = 0; i < n; i++) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 分配内存
newNode->data = elements[i]; // 存储数据
newNode->next = head; // 链接新节点到头部
head = newNode; // 更新头节点
}
}
```
3. 输出顺序表元素:
```c
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
```
4. 主函数中调用上述函数并比较输入和输出:
```c
int main() {
int n, input_data[] = {/* 输入的数组元素 */};
printf("Enter the length of the list and its elements: ");
scanf("%d", &n);
createList(n, input_data);
printList(head); // 打印顺序表
return 0;
}
```
在这个程序中,你需要在主函数里提供输入的序列长度和元素值,然后创建顺序表并检查输出是否与输入一致。
阅读全文
相关推荐
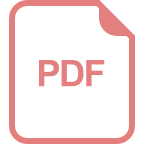
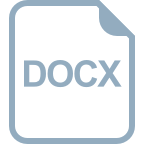
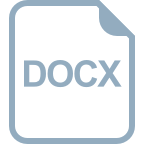
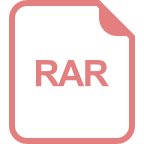
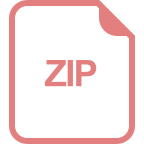
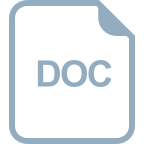
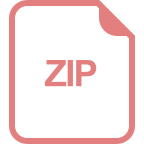
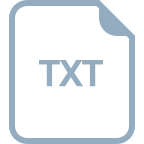
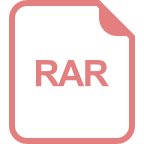
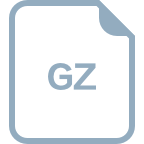
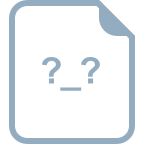
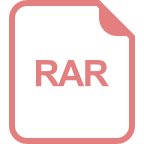
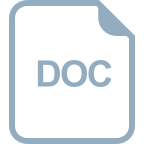