python formatf
时间: 2023-11-26 11:36:05 浏览: 73
Python中的格式化字符串可以使用两种方法:使用百分号(%)运算符和使用字符串的format()方法。
1. 使用百分号(%)运算符进行格式化:
```python
name = "John"
age = 25
print("My name is %s and I am %d years old." % (name, age))
```
输出:
```
My name is John and I am 25 years old.
```
2. 使用字符串的format()方法进行格式化:
```python
name = "John"
age = 25
print("My name is {} and I am {} years old.".format(name, age))
```
输出:
```
My name is John and I am 25 years old.
```
除了这两种方法,还有其他更高级的字符串格式化方式,如使用f字符串(f-strings):
```python
name = "John"
age = 25
print(f"My name is {name} and I am {age} years old.")
```
输出:
```
My name is John and I am 25 years old.
```
这些都是Python中常用的字符串格式化方法,你可以根据自己的需求选择使用其中的一种。
相关问题
python format 与 f
Python 中的 `format` 方法和格式化字符串字面量(通常称为 f-string)是两种常用的字符串格式化方法,它们允许我们以一种更加优雅和简洁的方式嵌入表达式的值。
1. `format` 方法:这是一种字符串格式化方法,它使用大括号 `{}` 作为占位符,并通过 `format` 方法的参数替换这些占位符。例如:
```python
name = "张三"
age = 30
formatted_string = "你好,{}。你的年龄是{}岁。".format(name, age)
```
在这个例子中,`{}` 会被 `format` 方法的参数替换,最终 `formatted_string` 的值为 "你好,张三。你的年龄是30岁。"。
2. f-string:这是在 Python 3.6 版本中引入的格式化字符串字面量的简写形式。它通过在字符串前加上字母 `f` 来实现,在字符串中可以直接嵌入变量或表达式,并使用花括号 `{}` 包围它们。例如:
```python
name = "李四"
age = 25
f_string = f"你好,{name}。你的年龄是{age}岁。"
```
在这里,变量 `name` 和 `age` 的值会直接嵌入到字符串中,`f_string` 的值为 "你好,李四。你的年龄是25岁。"。
f-string 的使用通常更加直观和简洁,它的执行速度也比传统的 `format` 方法快,因此在新版本的 Python 中,f-string 越来越受到开发者的青睐。
python format 简写 f
Python 中的 format() 方法提供了一种格式化字符串的方法,可以将变量值插入到字符串中。其中,format 简写 f 是一种比较新的方式,可以让代码更加简洁和易读。
使用 f 简写时,需要在字符串前面加上 f 或 F,表示这是一个格式化字符串。然后,在字符串中使用花括号 {} 表示要插入变量的位置,可以在花括号中添加变量名或表达式。
例如,如果有一个变量 name,其值为“张三”,则可以用 f 简写来构造一个字符串,如下所示:
```
name = "张三"
greeting = f"你好,{name}!"
print(greeting)
```
运行结果为:
```
你好,张三!
```
在上面的代码中,使用 f 简写可以轻松地将变量插入到字符串中,并且代码更加简洁和易读。同时,这种方式还支持各种格式化选项,可以更灵活地控制输出的格式。
总之,f 简写是 Python 中格式化字符串的一种简洁而强大的方式,可以让开发者更加高效地构造字符串。
阅读全文
相关推荐
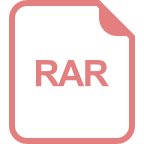
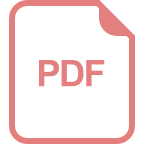
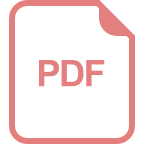










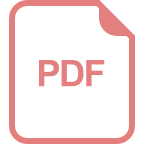
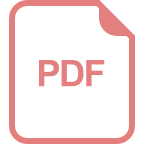
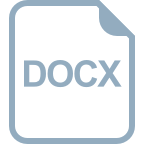
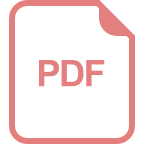