c语言判断一下2000年-2024年哪些年是闰年
时间: 2024-06-15 15:05:58 浏览: 203
在C语言中,可以使用条件语句和逻辑运算符来判断一个年份是否为闰年。根据闰年的定义,满足以下两个条件之一的年份是闰年:
1. 能被4整除但不能被100整除;
2. 能被400整除。
下面是一个示例代码,用于判断2000年到2024年之间的闰年:
```c
#include <stdio.h>
int main() {
int startYear = 2000;
int endYear = 2024;
printf("闰年列表:\n");
for (int year = startYear; year <= endYear; year++) {
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) {
printf("%d\n", year);
}
}
return 0;
}
```
运行以上代码,将输出2000年到2024年之间的闰年列表:
```
闰年列表:
2000
2004
2008
2012
2016
2020
2024
```
相关问题
C语言判断是闰年还是平年
在C语言中,判断闰年的规则是这样的:如果一个年份能被4整除,那么它是闰年。但是有例外,世纪年份(即能被100整除的年份)如果是闰年,还必须能够被400整除。例如,1900年不是闰年,而2000年是闰年。
以下是判断闰年的简单C语言函数:
```c
#include <stdbool.h>
// 判断是否为闰年的函数
bool is_leap_year(int year) {
if (year % 4 != 0) { // 如果不能被4整除,则直接返回false
return false;
}
if (year % 100 != 0) { // 如果能被4整除但不能被100整除,则是闰年
return true;
}
if (year % 400 != 0) { // 如果能被100整除但不能被400整除,则不是闰年
return false;
} else { // 如果能被400整除,那就是闰年
return true;
}
}
int main() {
int year = 2024; // 测试一下
if (is_leap_year(year)) {
printf("%d is a leap year.\n", year);
} else {
printf("%d is not a leap year.\n", year);
}
return 0;
}
```
用C语言判断年份是否为闰年
在C语言中,判断一个年份是否为闰年的规则有以下几种常见的标准:
- **普通闰年规则**:能被4整除的年份通常是闰年,除非它能被100整除但不能被400整除。例如,1900年不是闰年,因为它可以被100整除但不能被400整除;而2000年是闰年,因为它同时满足能被4和400整除的条件。
**代码示例**:
```c
#include <stdbool.h>
bool isLeapYear(int year) {
if (year % 4 != 0) { // 如果不是4的倍数,则不是闰年
return false;
}
else if (year % 100 == 0) { // 如果是100的倍数,看是否同时能被400整除
if (year % 400 == 0)
return true;
else
return false;
} else { // 否则是4的倍数且不是100的倍数,是闰年
return true;
}
}
int main() {
int year = 2024;
if (isLeapYear(year))
printf("%d is a leap year.\n", year);
else
printf("%d is not a leap year.\n", year);
return 0;
}
```
在这个例子中,`isLeapYear()` 函数接收一个整数作为年份参数,并返回一个布尔值,表示该年份是否为闰年。
阅读全文
相关推荐
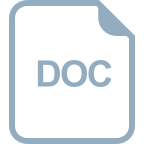
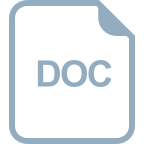
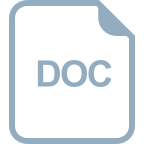
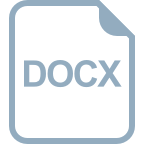
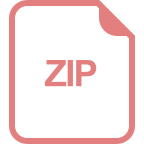
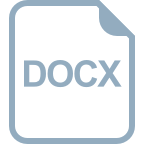










