C# 实现客户端从ftp服务器静默下载文件到指定路径
时间: 2024-10-20 22:07:18 浏览: 15
在C#中,你可以使用System.IO.FtpClient库来实现在FTP服务器上静默下载文件。下面是一个简单的示例,展示如何创建FTP连接、登录、查找文件并将其下载到本地特定路径:
```csharp
using System;
using System.IO;
using System.Net;
class Program
{
static void Main(string[] args)
{
string ftpServer = "your_ftp_server_address";
int ftpPort = 21; // FTP默认端口
string username = "your_username";
string password = "your_password";
string remoteFilePath = "/path/to/remote/file.txt";
string localFilePath = @"C:\Temp\downloaded_file.txt"; // 下载路径
try
{
using (FtpWebRequest request = (FtpWebRequest)WebRequest.Create($"ftp://{ftpServer}:{ftpPort}/{remoteFilePath}"))
{
request.Method = WebRequestMethods.Ftp.DownloadFile;
request.UseBinary = true;
request.Credentials = new NetworkCredential(username, password);
FtpWebResponse response = (FtpWebResponse)request.GetResponse();
using (FileStream fs = File.OpenWrite(localFilePath))
{
response.InputStream.CopyTo(fs);
Console.WriteLine($"File downloaded successfully to {localFilePath}");
}
}
}
catch (Exception ex)
{
Console.WriteLine($"Error occurred while downloading file: {ex.Message}");
}
Console.ReadLine();
}
}
```
在这个例子中,你需要替换`your_ftp_server_address`、`username`、`password`、`remoteFilePath`为你实际的FTP服务器信息和文件路径。这个程序会下载文件到本地指定的目录,并在控制台打印出成功消息。由于是在控制台上运行,所以可以认为是“静默”的下载过程。
阅读全文
相关推荐
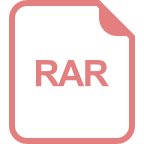
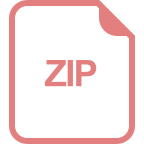
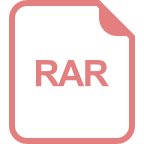
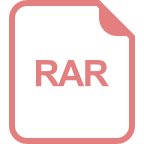
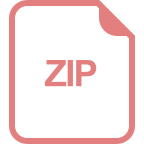
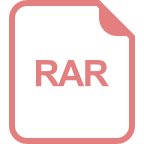
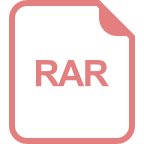
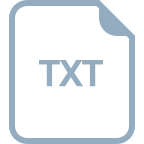
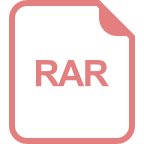
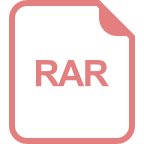
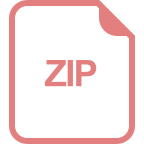
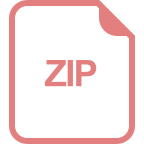
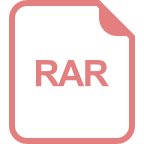