wpf textbox双向绑定
时间: 2025-01-07 19:12:47 浏览: 7
### WPF TextBox 控件的双向数据绑定
在 Windows Presentation Foundation (WPF) 中,`TextBox` 控件可以通过设置 `Binding` 的 `Mode` 属性为 `TwoWay` 来实现双向数据绑定。这使得用户界面中的更改可以立即反映到后台的数据模型中,反之亦然。
#### 创建 ViewModel 类
为了更好地管理应用程序的状态并支持 MVVM 架构,在此创建一个简单的 ViewModel 类来处理数据逻辑:
```csharp
using System.ComponentModel;
public class MainViewModel : INotifyPropertyChanged
{
private string _textBoxContent;
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
public string TextBoxContent
{
get => _textBoxContent;
set
{
if (_textBoxContent != value)
{
_textBoxContent = value;
OnPropertyChanged(nameof(TextBoxContent));
}
}
}
}
```
上述代码定义了一个名为 `MainViewModel` 的类,并实现了 `INotifyPropertyChanged` 接口以便通知 UI 更新[^1]。
#### XAML 部分配置
接下来是在 XAML 文件里声明 `TextBox` 并为其属性应用绑定表达式:
```xml
<Window x:Class="YourNamespace.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<Grid>
<!-- 设置 DataContext -->
<Window.DataContext>
<local:MainViewModel />
</Window.DataContext>
<!-- 绑定 Text 属性至 ViewModel 中的 TextBoxContent 属性 -->
<TextBox Width="200" Height="30" Margin="10"
Text="{Binding Path=TextBoxContent, Mode=TwoWay}" />
<!-- 显示当前文本框的内容 -->
<TextBlock VerticalAlignment="Center" HorizontalAlignment="Left" Margin="10,50,0,0">
Current Content: <Run Text="{Binding Path=TextBoxContent}"/>
</TextBlock>
</Grid>
</Window>
```
这里设置了 `DataContext` 为新实例化的 `MainViewModel` 对象;接着通过 `{Binding}` 表达式指定了 `TextBox.Text` 和 `TextBlock.Text` 应该与 `MainViewModel.TextBoxContent` 进行同步。
#### UpdateSourceTrigger 参数的作用
默认情况下,当失去焦点时才会触发源更新行为。如果希望每次键入字符都实时更新,则可以在 Binding 上指定 `UpdateSourceTrigger=PropertyChanged`:
```xml
<TextBox ... Text="{Binding Path=TextBoxContent, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}" />
```
这样做的好处是可以即时响应用户的每一个输入动作,而不需要等待控件失焦后再做处理。
阅读全文
相关推荐






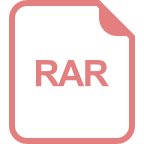









