我该如何将其转换成java代码?
时间: 2024-10-20 18:03:57 浏览: 6
将C++中的`gf28`类转换为Java代码需要考虑一些关键点,包括数据类型的转换、运算符重载的替代方法以及静态方法的实现。以下是将C++代码转换为Java代码的一个示例:
### Java版本的 `Gf28` 类
```java
public class Gf28 {
private byte value;
public Gf28() {
this.value = 0;
}
public Gf28(int arg) {
this.value = (byte) arg;
}
public Gf28(byte arg) {
this.value = arg;
}
// Overloaded methods for addition
public Gf28 add(Gf28 other) {
return new Gf28(this.value ^ other.value);
}
public Gf28 add(int other) {
return new Gf28(this.value ^ (byte) other);
}
// Overloaded methods for subtraction
public Gf28 subtract(Gf28 other) {
return new Gf28(this.value ^ other.value);
}
public Gf28 subtract(int other) {
return new Gf28(this.value ^ (byte) other);
}
// Overloaded methods for multiplication
public Gf28 multiply(Gf28 other) {
return new Gf28(multiply(this.value, other.value));
}
public Gf28 multiply(int other) {
return new Gf28(multiply(this.value, (byte) other));
}
// Overloaded methods for modulus
public Gf28 mod(Gf28 other) {
return new Gf28(modulo(this.value, other.value));
}
public Gf28 mod(int other) {
return new Gf28(modulo(this.value, (byte) other));
}
// Overloaded methods for division
public Gf28 divide(Gf28 other) {
return divide(this, other);
}
// Equality check
public boolean equals(Gf28 other) {
return this.value == other.value;
}
// Static methods
private static byte multiply(byte a, byte b) {
byte c = 0;
while (a != 0 && b != 0) {
if ((b & 1) != 0) c ^= a;
if ((a & 0x80) != 0) a = (byte) ((a << 1) ^ 0x11b);
else a <<= 1;
b >>>= 1;
}
return c;
}
private static Gf28 divide(Gf28 arg0, Gf28 arg1) {
int invArg;
for (invArg = 0; invArg < 255; invArg++) {
Gf28 c = arg1.multiply(invArg);
if (c.equals(new Gf28(1))) break;
}
return arg0.multiply(invArg);
}
private static byte modulo(byte a, byte b) {
if (b > a) return a;
else {
int c = 0;
while (msb(a) > msb(b)) {
b = (byte) (b << 1);
c++;
}
for (int c2 = 0; c2 < c; c2++) {
if (msb(b) == msb(a)) a = (byte) (a ^ b);
b = (byte) (b >>> 1);
}
return (byte) (a ^ b);
}
}
private static byte msb(int n) {
if (n == 0) return 0;
int msb = 0;
while (n != 0) {
n /= 2;
msb++;
}
return (byte) (1 << msb);
}
public byte xtime() {
return (byte) ((this.value << 1) ^ (((this.value >> 7) & 1) != 0 ? 0x1b : 0x00));
}
public byte x1time() {
return (byte) (this.value ^ xtime());
}
@Override
public String toString() {
return String.format("0x%02X", this.value);
}
public static void main(String[] args) {
Gf28 a = new Gf28(0xC8);
Gf28 b = new Gf28(0xC8);
Gf28 sum = a.add(b);
Gf28 diff = a.subtract(b);
Gf28 product = a.multiply(b);
Gf28 quotient = a.divide(b);
System.out.println("a: " + a);
System.out.println("b: " + b);
System.out.println("sum (a + b): " + sum);
System.out.println("difference (a - b): " + diff);
System.out.println("product (a * b): " + product);
System.out.println("quotient (a / b): " + quotient);
}
}
```
### 主要变化和注意事项
1. **数据类型**:C++中的`uint8_t`在Java中对应`byte`类型。
2. **运算符重载**:Java不支持运算符重载,因此使用方法来代替。
3. **构造函数**:Java中的构造函数与C++类似,但没有默认参数。
4. **静态方法**:将C++中的私有成员函数转换为Java中的静态方法。
5. **位操作**:Java中的位操作符与C++相同,但需要注意无符号右移`>>>`。
6. **字符串格式化**:使用`String.format`来进行十六进制输出。
通过这些调整,你可以将C++代码成功转换为Java代码,并保持功能的一致性。
阅读全文
相关推荐
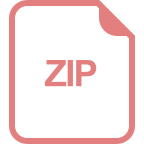
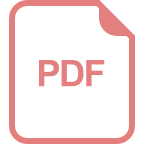
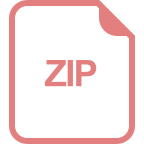
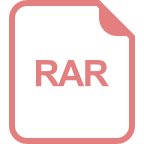
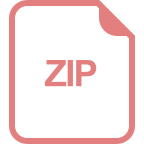
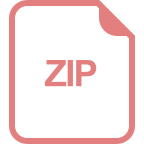
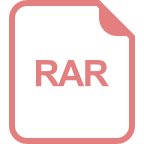
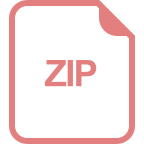
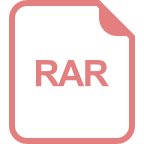
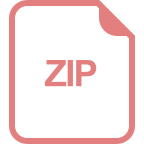
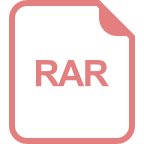
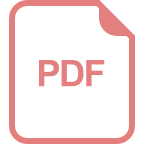
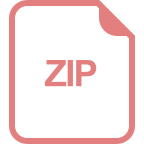
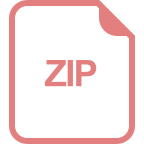
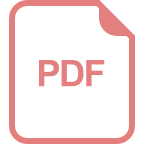
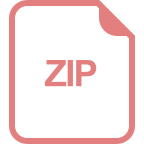
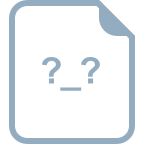