winform课程设计
时间: 2023-12-05 19:05:50 浏览: 60
为了回答这个问题,需要更多的信息。winform课程设计是一个非常广泛的主题,因此需要知道具体的要求和目标。以下是一些可能有用的信息和建议:
1. WinForms是一种Microsoft .NET框架下的GUI应用程序开发技术,它使用C#或VB.NET等编程语言。如果你不熟悉WinForms,可以先学习一些基础知识,例如如何创建窗体、添加控件、处理事件等。
2. 课程设计通常需要一个具体的主题或问题。你需要明确你的课程设计的主题是什么,例如一个学生管理系统、一个图书馆管理系统等等。
3. 数据库是许多课程设计的重要组成部分。你需要选择一个适合你的项目的数据库,例如SQL Server、MySQL等等,并学习如何使用它们。
4. 上传附件是一个常见的功能,可以使用C#的OpenFileDialog控件来实现。当用户点击上传按钮时,打开对话框,让用户选择文件,然后将文件上传到服务器或保存到本地。
5. 最后,你需要将所有的组件和功能整合到一个完整的应用程序中,并进行测试和调试。
以下是一个简单的WinForms课程设计的例子,它实现了一个简单的学生管理系统:
```csharp
// 创建一个窗体
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
}
// 添加学生按钮的事件处理程序
private void addButton_Click(object sender, EventArgs e)
{
// 从文本框中获取学生信息
string name = nameTextBox.Text;
int age = int.Parse(ageTextBox.Text);
string major = majorTextBox.Text;
// 将学生信息添加到数据库中
string connectionString = "Data Source=(local);Initial Catalog=StudentDB;Integrated Security=True";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
string sql = "INSERT INTO Students (Name, Age, Major) VALUES (@Name, @Age, @Major)";
using (SqlCommand command = new SqlCommand(sql, connection))
{
command.Parameters.AddWithValue("@Name", name);
command.Parameters.AddWithValue("@Age", age);
command.Parameters.AddWithValue("@Major", major);
command.ExecuteNonQuery();
}
}
// 更新学生列表
UpdateStudentList();
}
// 更新学生列表
private void UpdateStudentList()
{
// 从数据库中获取学生列表
string connectionString = "Data Source=(local);Initial Catalog=StudentDB;Integrated Security=True";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
string sql = "SELECT * FROM Students";
using (SqlCommand command = new SqlCommand(sql, connection))
{
using (SqlDataReader reader = command.ExecuteReader())
{
// 清空列表
studentListBox.Items.Clear();
// 添加学生到列表中
while (reader.Read())
{
string name = reader.GetString(1);
int age = reader.GetInt32(2);
string major = reader.GetString(3);
studentListBox.Items.Add(name + " (" + age + ") - " + major);
}
}
}
}
}
}
```
相关推荐
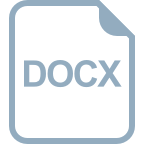
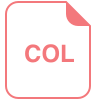













