创建 5 个 thread 结构体,为每个结构体的 tid 字段依次赋值为第二个参数数组 mytid 中的对应数值,并依次将其插入到第一个参数list 中,插入过程中要求使用自定义的字段 myelem 作为链表元素挂载到 list 中。
时间: 2024-11-27 14:10:00 浏览: 18
首先,你需要创建一个 Thread 结构体,包含 tid 和 myelem 字段。然后,你可以使用 C++ 的标准库如 `<thread>` 来创建线程,并通过 lambda 函数或成员函数指针将每个线程关联到对应的 tid。为了将线程安全地插入链表,通常需要考虑使用互斥锁(`std::mutex`)来同步操作。
```cpp
#include <vector>
#include <thread>
#include <mutex>
// 假设 Thread 结构体定义如下
struct Thread {
int tid;
std::string myelem; // 或其他适当的数据类型
};
// 假设我们有一个包含 tid 的 vector 和一个链表模板
std::vector<int> mytid = {1, 2, 3, 4, 5}; // 第二个参数数组
std::list<Thread*> list;
// 自定义的互斥锁
std::mutex mtx;
// 用于创建并插入线程的辅助函数
void insertThread(Thread* t) {
std::lock_guard<std::mutex> lock(mtx);
list.push_back(t);
}
int main() {
for (size_t i = 0; i < mytid.size(); ++i) {
Thread t{mytid[i], "Thread_" + std::to_string(i)}; // 设置 tid 和 myelem
// 使用 thread 对象和 insertThread 函数
std::thread worker(insertThread, &t); // 将线程和插入函数绑定
worker.join(); // 等待线程完成
}
return 0;
}
```
在这个例子中,`insertThread` 函数会在插入线程之前获取互斥锁,保证了并发环境下的数据一致性。每个新创建的线程 `worker` 都会将当前的 `Thread` 实例传递给 `insertThread`,并在主线程中等待其执行完毕。
阅读全文
相关推荐
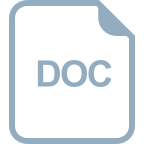
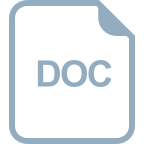
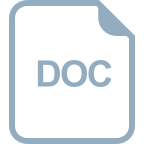
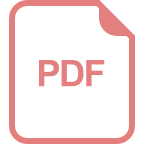
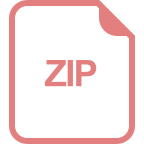
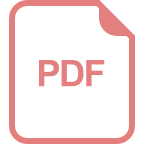
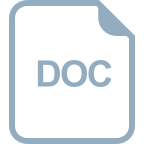
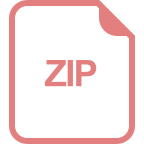
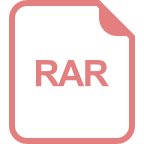
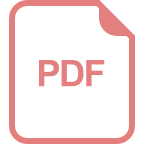
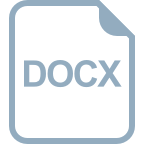
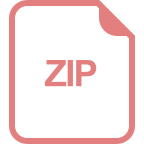
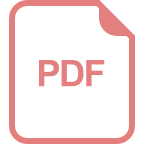
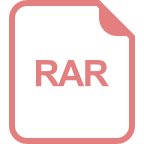
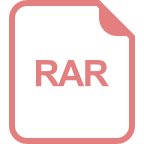
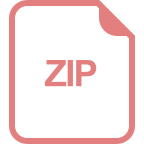
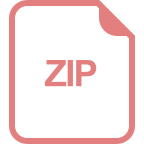