msp430f149内部定时器实现的实时时钟,可调节和可显示年月日时分秒,设置闹钟,用lcd
时间: 2024-02-02 13:01:22 浏览: 91
MSP430F149是一种微控制器,它具有内部定时器可以实现实时时钟的功能。可以通过编程来控制定时器的频率和计数器,从而实现可调节和可显示年月日时分秒的实时时钟功能。
要实现实时时钟功能,首先需要初始化定时器并设置其频率。可以根据实际需求选择适当的频率,然后通过定时器中断来记录时间的流逝并更新时钟显示。
在MSP430F149上集成一个LCD模块,可以用来显示年月日时分秒。通过编程将时钟信息转换成LCD显示的格式,并将其传输到LCD模块上进行显示。
另外,MSP430F149还可以设置闹钟功能。通过编程来设置闹钟的触发时间,一旦达到设定的时间,可以触发相应的中断,以实现闹钟的功能。
总体来说,要实现MSP430F149内部定时器实现的实时时钟功能,需要通过编程对定时器进行设置和控制,将时钟信息转换为LCD显示的格式,并设置闹钟功能。这样就可以实现一个能够调节和显示年月日时分秒,并带闹钟功能的实时时钟系统。
阅读全文
相关推荐
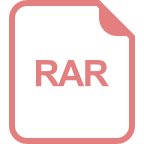
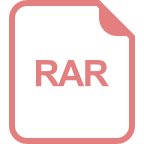
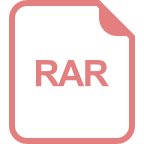
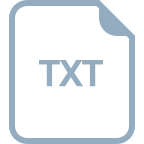
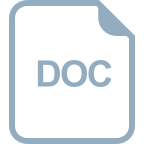
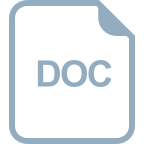
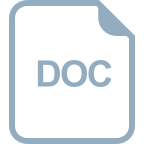
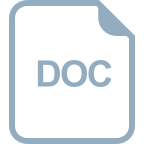
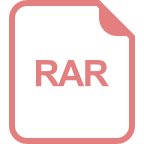
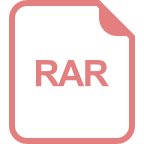
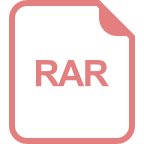
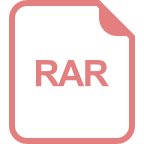
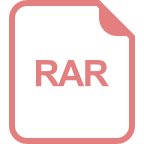
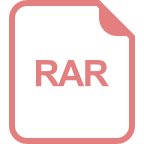