springboot整合 webflux调用chirpstack API
时间: 2023-12-27 22:01:49 浏览: 43
你可以使用Spring WebFlux来调用ChirpStack API。首先,你需要在你的Spring Boot项目中添加WebFlux的依赖。
在你的pom.xml文件中添加以下依赖:
```xml
<dependencies>
<!-- Spring Boot WebFlux -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
<!-- ChirpStack API client -->
<dependency>
<groupId>io.swagger</groupId>
<artifactId>swagger-annotations</artifactId>
<version>1.6.2</version>
</dependency>
</dependencies>
```
接下来,你需要创建一个ChirpStack API的客户端。你可以使用Swagger Codegen来生成一个基于API定义的Java客户端。
1. 在Swagger Codegen的官方网站上选择ChirpStack API的定义文件(通常是一个OpenAPI规范文件,如JSON或YAML格式)。
2. 使用Swagger Codegen生成Java客户端代码。你可以选择生成Jersey、OkHttp或其他HTTP客户端的代码。确保选择生成Java代码,并将其保存到你的项目中。
在你的Spring Boot应用程序中,创建一个用于调用ChirpStack API的服务类。这个服务类应该使用生成的ChirpStack API客户端来执行API调用。
```java
import io.swagger.client.ApiClient;
import io.swagger.client.ApiException;
import io.swagger.client.api.DefaultApi;
import io.swagger.client.model.Device;
import org.springframework.stereotype.Service;
@Service
public class ChirpStackService {
private final DefaultApi chirpStackApi;
public ChirpStackService() {
// 创建一个ChirpStack API客户端
ApiClient apiClient = new ApiClient();
apiClient.setBasePath("http://your-chirpstack-api-host");
this.chirpStackApi = new DefaultApi(apiClient);
}
public Device getDevice(String deviceId) throws ApiException {
// 调用ChirpStack API获取设备信息
return chirpStackApi.getDevice(deviceId);
}
}
```
在你的控制器类中,注入ChirpStackService并使用它来调用ChirpStack API。
```java
import io.swagger.client.model.Device;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/devices")
public class DeviceController {
private final ChirpStackService chirpStackService;
@Autowired
public DeviceController(ChirpStackService chirpStackService) {
this.chirpStackService = chirpStackService;
}
@GetMapping("/{deviceId}")
public Device getDevice(@PathVariable String deviceId) throws ApiException {
return chirpStackService.getDevice(deviceId);
}
}
```
这样,你就可以通过调用`GET /devices/{deviceId}`接口来获取ChirpStack设备的信息。
请注意,这只是一个基本的示例。你可能需要根据ChirpStack API的具体要求进行进一步的开发和调整。另外,确保在使用ChirpStack API时提供正确的API端点和身份验证信息。
相关推荐
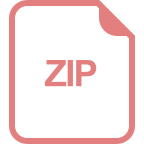
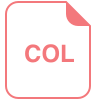
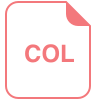
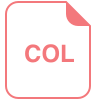
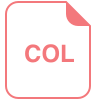
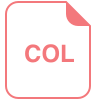









