请用C语言编程实现一个对顺序表进行基本操作的系统,主要包括表的创建、输入、查询、取值、插入、删除和输出等操作
时间: 2024-10-17 10:09:56 浏览: 26
在C语言中,我们可以使用结构体来表示顺序表,并通过函数来实现基本的操作。这里是一个简单的示例,定义了一个顺序表的结构以及几个常用的操作:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义顺序表节点结构
typedef struct Node {
int data; // 数据域
struct Node* next; // 指向下一个节点的指针
} ListNode;
// 初始化顺序表
ListNode* createList() {
return (ListNode*)malloc(sizeof(ListNode));
}
// 插入元素到列表尾部
void insert(ListNode** head, int value) {
ListNode* newNode = createList();
if (*head == NULL) {
*head = newNode;
} else {
ListNode* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
newNode->data = value;
newNode->next = NULL;
}
// 删除指定位置的元素
void delete(ListNode** head, int index) {
if (*head == NULL || index <= 0) return;
if (index == 1) { // 如果要删除的是头节点
ListNode* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
ListNode* current = *head;
for (int i = 1; i < index && current != NULL; i++) {
current = current->next;
}
if (current == NULL) return;
ListNode* temp = current->next;
current->next = temp->next;
free(temp);
}
// 查询元素是否存在
int search(ListNode* head, int value) {
ListNode* current = head;
while (current != NULL) {
if (current->data == value) {
return 1; // 找到了
}
current = current->next;
}
return 0; // 没找到
}
// 输出列表内容
void display(ListNode* head) {
ListNode* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
int main() {
ListNode* listHead = createList(); // 创建空列表
// ... 进行其他操作,如插入、删除、查询和显示
return 0;
}
```
阅读全文
相关推荐
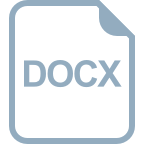
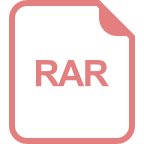
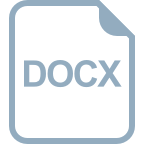





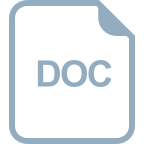
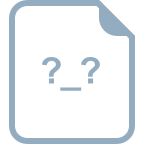
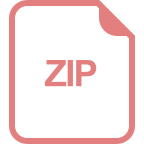
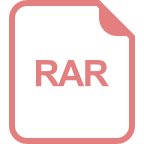
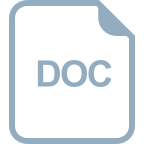
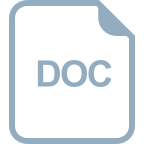
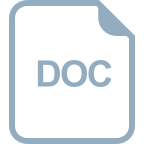
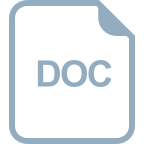
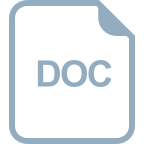