重点是维修回应界面,要能在正常修改表格数据后使数据库表中的数据也改变
时间: 2024-12-23 19:12:44 浏览: 8
### 维修回应界面关键点总结
1. **数据展示**:
- 使用 `SELECT * FROM RepairInfo` 查询语句获取所有维修记录。
- 将查询结果展示在一个表格中,方便物业查看和编辑。
2. **数据编辑**:
- 物业可以通过界面选择一行数据进行编辑。
- 编辑完成后,点击“编辑”按钮将修改后的数据保存到数据库。
3. **数据更新**:
- 在 `SqlServerDao` 类中定义 `updateRepair()` 方法,用于将编辑后的数据更新到数据库。
- 更新时,默认设置 `FromRepairFund` 为 1,表示维修费用由维修基金支付。
- 更新操作会触发 `UpdateMaintenanceFund` 触发器,自动扣除用户的维修费用。
4. **触发器逻辑**:
- `UpdateMaintenanceFund` 触发器在 `Amount` 字段更新时被激活。
- 触发器检查 `FromRepairFund` 是否为 1,如果是,则从用户的维护基金中扣除相应的维修费用。
5. **数据库连接**:
- 使用 JDBC 连接 SQL Server 数据库。
- 配置数据库连接参数,包括驱动、URL、用户名和密码。
6. **界面实现**:
- 使用 Java Swing 构建响应维修界面。
- 显示数据库表内容的表格控件(如 `JTable`)。
- 提供编辑和更新按钮,以及退出按钮。
### 核心代码示例
#### 数据库连接类 (`SqlServerDao.java`)
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class SqlServerDao {
private static final String JDBC_DRIVER = "com.microsoft.sqlserver.jdbc.SQLServerDriver";
private static final String DB_URL = "jdbc:sqlserver://127.0.0.1:1433;databaseName=ResidentialPropertyManagementSystem";
private static final String USER = "sa";
private static final String PASS = "zzy750906";
public static Connection getConnection() throws SQLException {
try {
Class.forName(JDBC_DRIVER);
System.out.println("Connecting to database...");
Connection conn = DriverManager.getConnection(DB_URL, USER, PASS);
System.out.println("Connected database successfully...");
return conn;
} catch (ClassNotFoundException e) {
e.printStackTrace();
throw new SQLException(e.getMessage());
}
}
public void updateRepair(int repairId, String buildingNo, String roomNo, String repairContent, LocalDate reportDate, LocalDate repairDate, double amount, boolean fromRepairFund, String repairPerson) {
// 实现更新逻辑
}
}
```
#### 响应维修界面 (`RepairResponseUI.java`)
```java
import javax.swing.*;
import javax.swing.table.DefaultTableModel;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.time.LocalDate;
public class RepairResponseUI extends JFrame {
private JTable table;
private DefaultTableModel model;
public RepairResponseUI() {
setTitle("维修回应界面");
setSize(800, 600);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
model = new DefaultTableModel();
table = new JTable(model);
model.addColumn("楼号");
model.addColumn("房号");
model.addColumn("维修内容");
model.addColumn("申请日期");
model.addColumn("维修日期");
model.addColumn("维修金额");
model.addColumn("是否已支出");
model.addColumn("维修人员");
model.addColumn("维修ID");
loadTableData();
JButton editButton = new JButton("编辑");
editButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int selectedRow = table.getSelectedRow();
if (selectedRow != -1) {
// 获取选中的行数据
String buildingNo = (String) table.getValueAt(selectedRow, 0);
String roomNo = (String) table.getValueAt(selectedRow, 1);
String repairContent = (String) table.getValueAt(selectedRow, 2);
LocalDate reportDate = LocalDate.parse((String) table.getValueAt(selectedRow, 3));
LocalDate repairDate = LocalDate.parse((String) table.getValueAt(selectedRow, 4));
double amount = Double.parseDouble((String) table.getValueAt(selectedRow, 5));
boolean fromRepairFund = Boolean.parseBoolean((String) table.getValueAt(selectedRow, 6));
String repairPerson = (String) table.getValueAt(selectedRow, 7);
int repairId = Integer.parseInt((String) table.getValueAt(selectedRow, 8));
// 调用更新方法
SqlServerDao dao = new SqlServerDao();
dao.updateRepair(repairId, buildingNo, roomNo, repairContent, reportDate, repairDate, amount, fromRepairFund, repairPerson);
// 刷新表格
loadTableData();
}
}
});
JButton exitButton = new JButton("退出");
exitButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
dispose();
new PropertyManagementUI().setVisible(true);
}
});
add(new JScrollPane(table));
JPanel buttonPanel = new JPanel();
buttonPanel.add(editButton);
buttonPanel.add(exitButton);
add(buttonPanel, "South");
}
private void loadTableData() {
model.setRowCount(0);
try (Connection conn = SqlServerDao.getConnection()) {
PreparedStatement ps = conn.prepareStatement("SELECT * FROM RepairInfo");
ResultSet rs = ps.executeQuery();
while (rs.next()) {
Object[] row = new Object[]{
rs.getString("BuildingNO"),
rs.getString("RoomNO"),
rs.getString("RepairContent"),
rs.getDate("ReportDate").toLocalDate(),
rs.getDate("RepairDate") == null ? "" : rs.getDate("RepairDate").toLocalDate(),
rs.getDouble("Amount"),
rs.getBoolean("FromRepairFund"),
rs.getString("RepairPerson"),
rs.getInt("RepairID")
};
model.addRow(row);
}
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
new RepairResponseUI().setVisible(true);
}
}
```
通过以上步骤和代码示例,可以实现一个功能完整的维修回应界面,支持数据展示、编辑和更新。
阅读全文
相关推荐
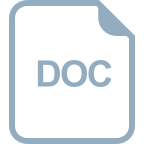
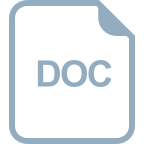
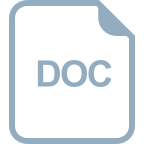
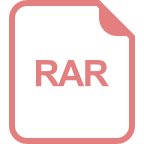
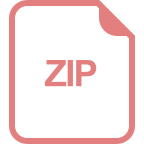
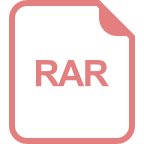
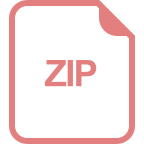
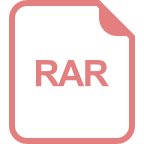
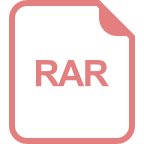
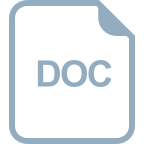
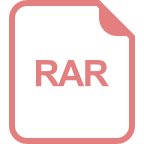
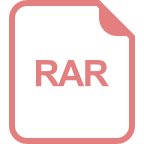
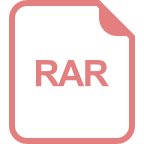
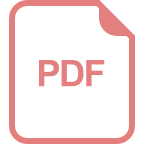
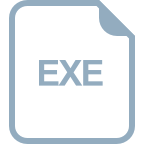
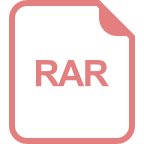
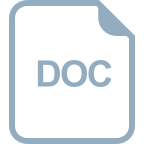
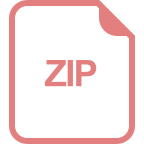
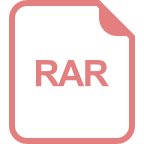