vue3在父组件中点击提交时,获取到子组件中的数据并在父组件中对该数据进行校验
时间: 2024-10-18 17:23:49 浏览: 7
在Vue3中,当你想要在父组件通过点击事件从子组件获取并验证数据时,可以采用以下步骤:
1. **定义父子通信**:
- 使用Vuex(状态管理库)存储全局共享的状态,包括子组件的数据。如果数据比较复杂,不适合直接传递。
- 或者,使用`ref`或` reactive`来创建计算属性,在子组件中暴露必要的数据给父组件。
```javascript
// 父组件中,引入store.js或其他状态管理工具
import { mapState } from 'vuex';
export default {
computed: {
...mapState('yourModule', ['childData']),
},
};
```
2. **在子组件中触发数据改变**:
当子组件需要更新数据时,使用`emit`自定义事件通知父组件。
```vue
<template>
<button @click="updateChildData">更新数据</button>
</template>
<script setup>
const updateChildData = () => {
// 更新子组件数据...
this.$emit('childDataUpdated', newData);
};
</script>
```
3. **父组件监听子组件事件**:
在父组件的模板中绑定一个处理事件的函数,当接收到`childDataUpdated`事件时,对数据进行校验。
```html
<template>
<button @click="validateData">提交</button>
<child-component :data.sync="childData" @childDataUpdated="handleChildDataUpdate"></child-component>
</template>
<script>
// ...
methods: {
handleChildDataUpdate(newData) {
if (validateYourData(newData)) {
// 数据校验通过,这里做进一步操作
}
},
validateYourData(data) {
// 根据需求编写数据验证规则
},
}
</script>
```
阅读全文
相关推荐
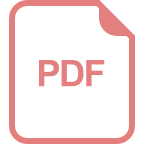
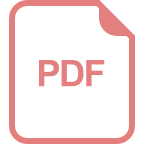
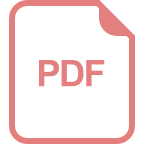















