生成一段爬虫代码,要求能够爬取谷歌网站的图片
时间: 2024-10-16 11:04:34 浏览: 22
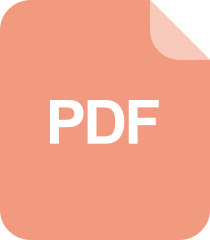
使用PyV8在Python爬虫中执行js代码
编写一个简单的Python爬虫来抓取Google网站的图片,我们将使用requests库来获取HTML内容,然后BeautifulSoup解析它来提取图片链接,最后使用os模块保存图片到本地。由于Google有反爬机制,并且直接爬取大量图片可能会违反其服务条款,所以这里仅示例如何抓取搜索结果页面的第一张图片:
```python
import requests
from bs4 import BeautifulSoup
import os
def get_google_image(search_term):
# 设置请求头,模拟浏览器访问
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
# Google图片搜索API地址
base_url = "https://www.google.com/searchbyimage?image_url="
google_search_url = f"https://www.google.com/search?q={search_term}&source=lnms&tbm=isch"
# 发送GET请求获取HTML
response = requests.get(google_search_url, headers=headers)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
# 查找第一个图片缩略图元素
img_element = soup.find('div', class_='rg_i Q4LuWd')
if img_element:
# 提取图片URL
img_url = img_element.find('img')['src']
# 保存图片到本地
save_path = f"{search_term}_image.jpg"
with open(save_path, 'wb') as img_file:
img_request = requests.get(img_url, stream=True)
for chunk in img_request.iter_content(chunk_size=1024):
if chunk:
img_file.write(chunk)
else:
print("No image found for the search term.")
else:
print(f"Failed to fetch page. Status code: {response.status_code}")
# 示例:爬取“猫”的第一张图片
get_google_image("猫")
```
注意:这个例子只能抓取搜索结果页面的图片,而Google的图片搜索结果包含多个页,如果你想获取更多图片,你需要循环发送请求到下一页。此外,频繁抓取Google图片可能会被视为滥用,建议只用于学习用途。
阅读全文
相关推荐
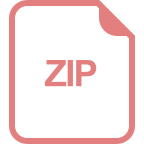
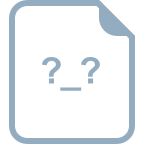
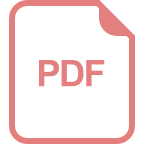
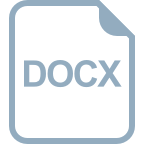
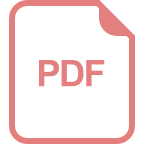
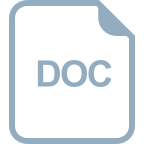
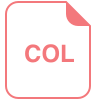
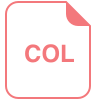
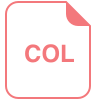
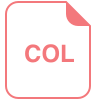
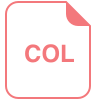
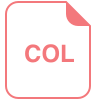
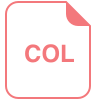
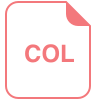
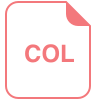
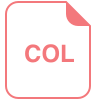
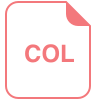

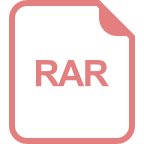