如何使用Python实现具有200次迭代限制,初始速度范围在0到1之间的多目标粒子群优化算法,并在该算法中找到帕累托解?请参考文档提供的具体内容进行代码实现。
时间: 2024-12-19 21:26:08 浏览: 25
为了使用Python实现具有200次迭代限制且初始速度范围在0到1之间的多目标粒子群优化(Multi-objective Particle Swarm Optimization, MOPSO),并基于文档提供的具体背景和目标寻找帕累托最优解,可以遵循以下步骤:
### 步骤1: 导入必要的库
```python
import numpy as np
from pymoo.algorithms.moo.pso import PSO
from pymoo.core.problem import ElementwiseProblem
from pymoo.optimize import minimize
from pymoo.visualization.scatter import Scatter
```
### 步骤2: 定义问题
根据文档描述,我们需要定义一个优化问题,该问题旨在最小化两种目标:总成本和碳排放。我们还需要定义决策变量、约束条件以及目标函数的具体形式。
```python
class PesticideWasteRecycling(ElementwiseProblem):
def __init__(self):
super().__init__(n_var=20, # 假设有20个决策变量
n_obj=2, # 两个目标:成本最小化和碳排放最小化
n_constr=0,
xl=np.zeros(20), # 最小边界
xu=np.ones(20)) # 最大边界
def _evaluate(self, x, out, *args, **kwargs):
# 这里需要根据文档中的公式计算成本和碳排放
cost = ... # 计算成本
carbon_emission = ... # 计算碳排放
out["F"] = [cost, carbon_emission]
```
### 步骤3: 实现具体的成本和碳排放计算
这部分需要根据文档中的公式来实现。这里提供一个简化的例子:
```python
def calculate_cost(x):
fixed_costs = sum([150000 if xi > 0.5 else 0 for xi in x[:10]]) + sum([300000 if xi > 0.5 else 0 for xi in x[10:]])
variable_costs = sum([65 * xi for xi in x[:10]]) + sum([200 * xi for xi in x[10:]])
transport_costs = 5.5 * sum([xi * 100 for xi in x]) # 假设平均运输距离为100公里
return fixed_costs + variable_costs + transport_costs
def calculate_carbon_emission(x):
emission_from_operations = sum([7.4 * xi for xi in x[:10]]) + sum([102 * xi for xi in x[10:]])
emission_from_transport = 0.35 * sum([xi * 100 for xi in x]) # 假设平均运输距离为100公里
return emission_from_operations + emission_from_transport
```
将这些函数集成到`_evaluate`方法中:
```python
class PesticideWasteRecycling(ElementwiseProblem):
def __init__(self):
super().__init__(n_var=20, # 假设有20个决策变量
n_obj=2, # 两个目标:成本最小化和碳排放最小化
n_constr=0,
xl=np.zeros(20), # 最小边界
xu=np.ones(20)) # 最大边界
def _evaluate(self, x, out, *args, **kwargs):
cost = calculate_cost(x)
carbon_emission = calculate_carbon_emission(x)
out["F"] = [cost, carbon_emission]
```
### 步骤4: 初始化PSO算法并运行
```python
algorithm = PSO(pop_size=100,
speed_range=[0, 1], # 初始速度范围
max_velocity=1, # 最大速度
min_velocity=0)
res = minimize(PesticideWasteRecycling(),
algorithm,
('n_gen', 200), # 200次迭代
seed=1,
verbose=True)
print("Best solution found: \nX = %s\nF = %s" % (res.X, res.F))
```
### 步骤5: 可视化结果
```python
plot = Scatter()
plot.add(res.F, color="red")
plot.show()
```
以上代码实现了多目标粒子群优化算法,用于解决农药包装废弃物回收物流网络的问题,并找到了帕累托最优解。你可以根据实际需求调整决策变量的数量、目标函数的具体实现以及其他参数。
阅读全文
相关推荐









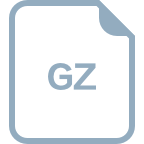







