我现在有sip服务器和信令服务器,如何实现浏览器端连接sip服务器进行通话,后端使用Java,将相关前后端代码写出来
时间: 2024-10-24 19:14:24 浏览: 11
要在浏览器端通过SIP服务器进行通话,并利用Java作为后端支持,你需要做的是设置WebRTC(实时通信)环境,结合SIP(Session Initiation Protocol)。这里是一个简化的步骤概述:
1. **前端(JavaScript + WebRTC)**:
- **安装库**: 使用`Simple-Jingle`或`js-sip-webrtc`这样的库,它们集成了SIP和WebRTC功能。
```javascript
// 引入依赖
const JINGLE = require('simple-jingle');
// 创建RTCPeerConnection实例
const pc = new RTCPeerConnection();
// 当接收到offer时处理SIP邀请
pc.onicecandidate = (event) => {
if (event.candidate) {
// 发送候选到SIP服务器
sendCandidateToServer(event.candidate);
}
};
function sendCandidateToServer(candidate) {
fetch('/api/sip/call', {
method: 'POST',
body: JSON.stringify({ candidate: candidate }),
headers: { 'Content-Type': 'application/json' },
})
.then(response => response.json())
.catch(err => console.error('Failed to send candidate:', err));
}
// 更多的处理过程,如接听offer、发送answer等
```
2. **后端(Java + SIP组件)**:
- **SIP服务**: 使用开源库如`jain-sip`或者商业解决方案(如Twilio Programmable Voice),处理SIP请求和响应。
```java
// 示例使用JAIN-SIP
import org.jitsi.net.xmpp.sip.JitsiSipAccountManager;
import org.jivesoftware.smackx.si.SIProfile;
// 初始化SIP账号管理器
JitsiSipAccountManager accountManager = new JitsiSipAccountManager();
SIProfile sipProfile = ...; // 配置SIP Profile
// 接收并处理来自前端的SIP候选者
@PostMapping("/api/sip/call")
public void receiveCandidate(@RequestBody CandidateRequest request) {
String candidate = request.getCandidate();
accountManager.addIceCandidate(sipProfile, candidate);
}
```
- **处理呼叫和媒体流**:
- 调用SIP服务器发起INVITE请求
- 管理会话状态和媒体传输。
注意这只是一个基本示例,实际项目中还需要考虑错误处理、认证、以及网络状况等因素。另外,确保服务器配置了正确的SIP域名、注册信息和防火墙规则。
阅读全文
相关推荐
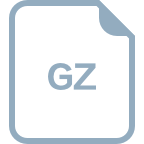
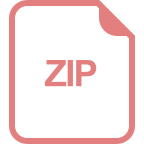
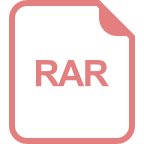
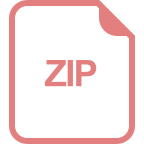
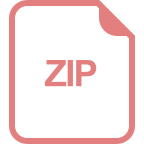
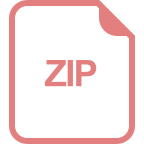
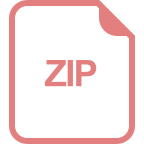
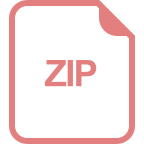
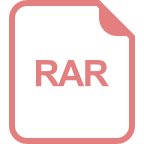
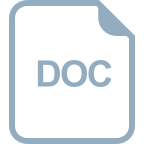
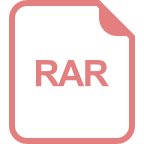
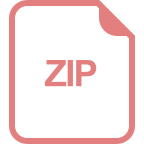
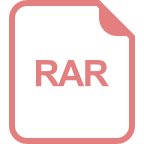
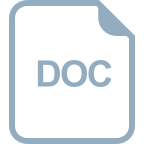



