qstring 转换为std::string
时间: 2024-09-19 22:00:44 浏览: 33
`QString` 是 Qt 库中的字符串类型,而 `std::string` 是 C++ 标准库里的字符串类型。如果你想从 `QString` 转换成 `std::string`,你可以使用 `Qt` 提供的一些工具函数。
如果你有 `QString str`,转换过程通常如下:
```cpp
#include <QString>
#include <string>
#include <QtWidgets/QApplication> // 如果你在使用 GUI 库,可能需要这个头文件
std::string QStringToStdString(const QString& qstr) {
return qstr.toStdString(); // 使用 .toStdString() 函数进行转换
}
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QString qtStr = "Hello, World!";
std::string str = QStringToStdString(qtStr);
// 现在 str 就包含了原始的 "Hello, World!" 字符串
// 可以进一步处理 std::string
return app.exec();
}
```
相关问题
利用std::codecvt_utf8将QString转换为std::string
在C++中,`std::codecvt_utf8`是一个用于字符集转换的工具,特别是在处理Unicode字符串时。如果你有一个Qt框架中的QString对象,它代表了UTF-8编码的宽字符序列,你可以通过`QByteArray`和`std::wstring_convert`结合`std::codecvt_utf8`将其转换成标准库的`std::string`,步骤如下:
1. 首先,包含必要的头文件:
```cpp
#include <QtWidgets/QApplication>
#include <QByteArray>
#include <locale>
#include <codecvt>
#include <string>
```
2. 创建一个`QByteArray`,通常从QString获取:
```cpp
QString utf8String = "你好,世界";
QByteArray utf8Bytes(utf8String.toUtf8());
```
3. 使用`std::wstring_convert`和`std::codecvt_utf8`进行转换:
```cpp
std::wstring_convert<std::codecvt_utf8<char>> converter;
std::string str = converter.from_bytes(utf8Bytes);
```
这将会把宽字符数组转换为对应的ASCII字符串。
Qstring转成std::string
要将QString转换为std::string,可以使用toStdString()函数。这个函数是QString类的成员函数,它将QString对象转换为std::string对象。下面是一个示例代码:
```cpp
QString qstr = "Hello, World!";
std::string str = qstr.toStdString();
```
在上面的代码中,我们将QString对象qstr转换为std::string对象str。现在,str就包含了与qstr相同的字符串内容。
需要注意的是,QString和std::string之间的转换可能会导致编码问题。QString使用的是Unicode编码,而std::string通常使用的是ASCII编码或UTF-8编码。因此,在进行转换时,需要确保编码方式的兼容性。
相关推荐
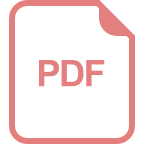
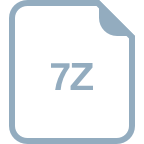
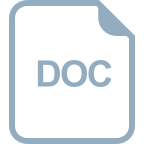












