python连接数据库创建表
时间: 2024-06-19 22:01:35 浏览: 8
在Python中,使用SQLAlchemy或pymysql等库连接数据库并创建表是非常常见的任务。这里我们以SQLite(内置数据库)和SQLAlchemy为例来说明,其他如MySQL、PostgreSQL等数据库略有不同但基本原理相似。
**使用SQLite和SQLAlchemy:**
首先,你需要安装SQLAlchemy库(如果还没有安装):
```bash
pip install sqlalchemy
```
然后,你可以创建一个SQLite连接并定义表结构:
```python
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
# 创建基础模型
Base = declarative_base()
# 定义表名和字段
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String(50), nullable=False)
email = Column(String(120), unique=True)
# 创建数据库引擎
engine = create_engine('sqlite:///example.db')
# 创建表
Base.metadata.create_all(engine)
```
**使用pymysql连接MySQL:**
首先,安装pymysql库:
```bash
pip install pymysql
```
然后使用如下代码创建连接并创建表:
```python
import pymysql
# 创建连接
connection = pymysql.connect(host='localhost', user='your_username', password='your_password', db='your_database')
# 创建游标
cursor = connection.cursor()
# 定义SQL语句
create_table_query = """
CREATE TABLE IF NOT EXISTS users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(50) NOT NULL,
email VARCHAR(120) UNIQUE
)
"""
# 执行SQL
cursor.execute(create_table_query)
connection.commit()
# 关闭游标和连接
cursor.close()
connection.close()
```
相关推荐
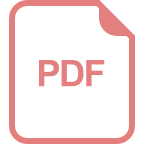
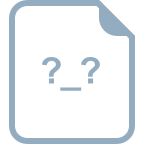
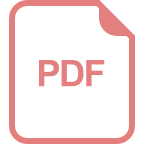
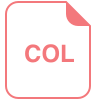
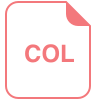
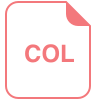
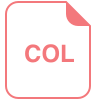
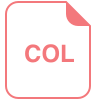









