C#程序代码如何通过查询一个结果集,遍历执行存储过程
时间: 2024-09-09 08:06:49 浏览: 56
在C#中,要通过查询结果集遍历执行存储过程,你可以使用ADO.NET(Active Directory Object Model.NET),特别是SqlCommand和SqlDataReader对象。以下是一个简单的示例:
```csharp
using System;
using System.Data.SqlClient;
public void ExecuteStoredProcedure()
{
string connectionString = "your_connection_string_here";
string storedProcedureName = "your_stored_procedure_name";
using (SqlConnection connection = new SqlConnection(connectionString))
{
// 创建SqlCommand对象并设置存储过程名
SqlCommand command = new SqlCommand(storedProcedureName, connection);
try
{
// 打开数据库连接
connection.Open();
// 如果存储过程返回结果集,可以使用ExecuteReader方法
SqlDataReader reader = command.ExecuteReader(CommandBehavior.CloseConnection);
// 使用while循环遍历结果集
while (reader.Read())
{
// 访问数据列
string column1Value = reader.GetString(0);
int column2Value = reader.GetInt32(1);
Console.WriteLine($"Column 1: {column1Value}, Column 2: {column2Value}");
}
// 关闭读取器,释放资源
reader.Close();
}
catch (Exception ex)
{
Console.WriteLine("Error executing stored procedure: " + ex.Message);
}
}
}
```
在这个例子中,你需要将`connection_string_here`替换为你实际的数据库连接字符串,并提供存储过程的名称。`GetString()`和`GetInt32()`是用来获取结果集中特定列值的方法。
阅读全文
相关推荐



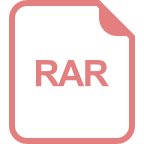

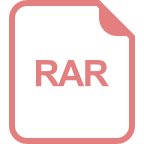

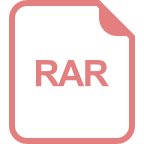

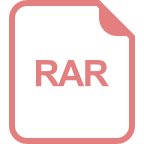

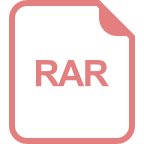






