vue3父调用子组件的值
时间: 2023-11-03 15:57:00 浏览: 46
在Vue3中,父组件可以通过使用ref来获得子组件的实例,在获得实例后,可以通过实例来访问子组件的数据和方法。在你提供的代码中,父组件使用ref指令关联了子组件,并给其起了一个名字叫做childComp。然后,在点击事件中,通过childComp.value来访问子组件的数据和方法。
所以,父组件调用子组件的值的代码可以这样写:
let childValue = childComp.value.state.value;
相关问题
vue父组件调用子组件值
在Vue中,父组件可以通过props属性将数据传递给子组件,然后子组件可以通过props接收父组件传递过来的值。这样子组件就可以在自己的模板中使用这些值。
下面是一个示例,展示了父组件如何调用子组件的值:
```html<!-- 父组件 -->
<template>
<div>
<p>父组件的值:{{ parentValue }}</p>
<child-component :child-value="parentValue"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent },
data() {
return {
parentValue: '父组件的值'
};
}
};
</script>
```
```html<!-- 子组件 -->
<template>
<div>
<p>子组件的值:{{ childValue }}</p>
</div>
</template>
<script>
export default {
props: ['childValue']
};
</script>
```
在上面的示例中,父组件通过props属性将`parentValue`的值传递给子组件,并且在子组件中使用`childValue`来接收这个值。然后子组件就可以在自己的模板中使用`childValue`来展示父组件传递过来的值。
注意:父组件通过`:`语法来绑定props属性,即`:child-value="parentValue"`。这样子组件才能正确接收父组件传递过来的值。
vue3父调用子组件方法
在 Vue 3 中,父组件可以通过 `ref` 或 `reactive` 创建一个引用,然后将其传递给子组件,从而可以调用子组件的方法。下面是一个示例:
在父组件中:
```vue
<template>
<div>
<button @click="callChildMethod">调用子组件方法</button>
<child-component :childRef="childRef"></child-component>
</div>
</template>
<script>
import { ref } from 'vue';
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
setup() {
// 创建一个 ref 引用
const childRef = ref(null);
// 父组件调用子组件方法
const callChildMethod = () => {
if (childRef.value) {
childRef.value.childMethod();
}
};
return {
childRef,
callChildMethod
};
}
};
</script>
```
在子组件中:
```vue
<template>
<div>
<p>子组件</p>
</div>
</template>
<script>
export default {
setup(props) {
// 子组件方法
const childMethod = () => {
console.log('子组件方法被调用');
};
return {
childMethod
};
}
};
</script>
```
在上面的示例中,父组件通过 `ref` 创建了一个引用 `childRef`,然后将其传递给子组件 `ChildComponent`。父组件中的 `callChildMethod` 方法可以通过 `childRef.value` 来访问子组件的方法 `childMethod`,从而实现了父组件调用子组件方法的功能。
相关推荐
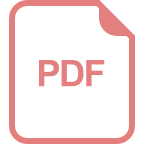












