用c++实现一个程序 功能:用户输入文本,程序检查文本中的拼写错误,并给出建议。
时间: 2024-09-17 07:06:21 浏览: 64
在C++中实现一个简单的拼写检查程序,可以使用第三方库如`levenshtein_distance`来计算单词间的编辑距离,然后通过比较用户输入和预定义字典中的单词来进行错误检测。以下是一个简化的示例,假设我们使用一个静态字符串数组作为字典:
```cpp
#include <iostream>
#include <string>
#include <unordered_map>
#include "distance.h" // 假设你已经包含了levenshtein_distance库
// 预定义字典
std::unordered_map<std::string, std::string> dictionary = {
{"hello", "hi"},
{"world", "world"},
{"example", "instance"}
};
int main() {
std::string input;
std::cout << "请输入文本: ";
getline(std::cin, input);
for (const auto &word : input.split(" ")) { // 分割单词
if (dictionary.find(word) == dictionary.end()) {
int minDistance = INT_MAX;
std::string suggestion;
// 检查字典中距离最短的单词
for (const auto &entry : dictionary) {
int dist = levenshtein_distance(entry.first, word);
if (dist < minDistance) {
minDistance = dist;
suggestion = entry.second;
}
}
if (minDistance > 0) {
std::cout << "错误单词: " << word << ", 建议: " << suggestion << std::endl;
}
} else {
std::cout << "单词: " << word << " 正确" << std::endl;
}
}
return 0;
}
阅读全文
相关推荐









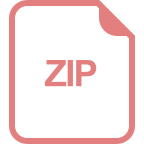

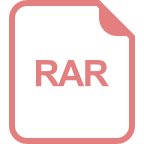





