serializable序列化
时间: 2023-12-09 10:04:21 浏览: 85
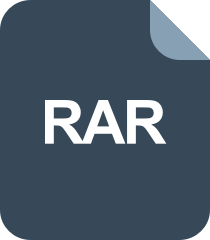
Serializable序列化
序列化是将对象转换为字节流的过程,以便可以将其存储在文件中、通过网络传输或在内存中进行持久化。在Java中,可以使用Serializable接口来实现对象的序列化和反序列化。
当一个类实现了Serializable接口时,它表示该类的对象可以被序列化。序列化的过程是将对象转换为一系列字节,以便可以将其保存到磁盘文件或通过网络传输。反序列化则是将字节流重新转换为对象。
要使一个类可序列化,需要遵循以下几个步骤:
1. 实现Serializable接口。
2. 如果类的实例变量引用了其他可序列化的对象,则这些对象也必须实现Serializable接口。
3. 为不希望被序列化的实例变量添加transient关键字。
以下是一个示例代码,演示了如何使用Serializable接口进行对象的序列化和反序列化:
```java
import java.io.*;
class MyClass implements Serializable {
private int intValue;
private String stringValue;
public MyClass(int intValue, String stringValue) {
this.intValue = intValue;
this.stringValue = stringValue;
}
// 省略其他代码...
public static void main(String[] args) {
MyClass object = new MyClass(10, "Hello");
// 序列化对象
try {
FileOutputStream fileOut = new FileOutputStream("object.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(object);
out.close();
fileOut.close();
System.out.println("对象已序列化");
} catch (IOException e) {
e.printStackTrace();
}
// 反序列化对象
try {
FileInputStream fileIn = new FileInputStream("object.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
MyClass deserializedObject = (MyClass) in.readObject();
in.close();
fileIn.close();
System.out.println("对象已反序列化");
System.out.println("intValue: " + deserializedObject.intValue);
System.out.println("stringValue: " + deserializedObject.stringValue);
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐
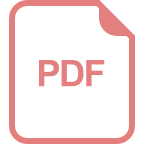
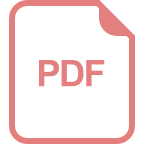















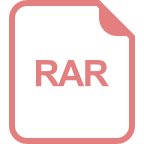