写一篇基于TensorFlow框架下VGG-16实现手写数字识别的代码 本实验用的手写数字数据集来自全班同学手写的数字数据集。
时间: 2024-12-10 17:34:58 浏览: 6
在TensorFlow框架下使用VGG-16实现手写数字识别需要经过以下几个步骤,这里提供一个简化的Python代码示例。首先,确保已经安装了必要的库,如`tensorflow`, `keras`, 和 `numpy`。我们将使用Keras作为高级API构建模型。
```python
# 导入所需的库
import tensorflow as tf
from tensorflow.keras.applications.vgg16 import VGG16, preprocess_input
from tensorflow.keras.layers import Dense, Flatten
from tensorflow.keras.models import Model
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# 加载预训练的VGG16模型,去掉顶部的分类层
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(64, 64, 3))
# 定义新的分类层
x = base_model.output
x = Flatten()(x)
predictions = Dense(10, activation='softmax')(x) # 10代表数字0-9,可以根据实际数据调整
# 创建新的模型,保留VGG16的所有卷积层
model = Model(inputs=base_model.input, outputs=predictions)
# 冻结基础模型的权重
for layer in base_model.layers:
layer.trainable = False
# 编译模型
model.compile(optimizer=Adam(lr=0.0001), loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# 数据预处理
train_datagen = ImageDataGenerator(preprocessing_function=preprocess_input, rescale=1./255)
test_datagen = ImageDataGenerator(preprocess_input, rescale=1./255)
# 加载手写数字数据集
train_generator = train_datagen.flow_from_directory('path_to_train_data',
target_size=(64, 64),
batch_size=32,
class_mode='sparse')
validation_generator = test_datagen.flow_from_directory('path_to_validation_data',
target_size=(64, 64),
batch_size=32,
class_mode='sparse')
# 训练模型
history = model.fit(train_generator,
steps_per_epoch=len(train_generator),
epochs=10, # 根据实际情况调整训练轮数
validation_data=validation_generator,
validation_steps=len(validation_generator))
# 打印一些关于模型性能的信息
print("Training accuracy:", history.history['accuracy'][-1])
print("Validation accuracy:", history.history['val_accuracy'][-1])
# 相关问题--
1. 这段代码中为什么要冻结VGG16的基础模型?
2. 使用ImageDataGenerator的作用是什么?
3. 如何调整模型结构以适应更大尺寸的手写数字图片?
阅读全文
相关推荐
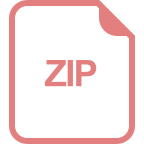
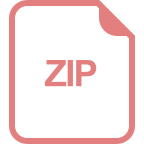
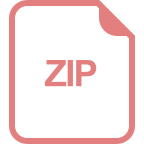
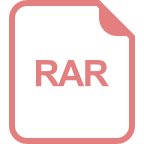
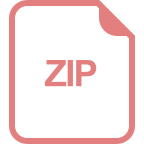
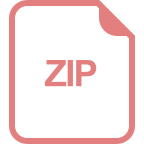
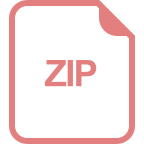
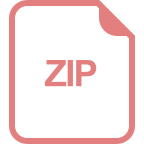
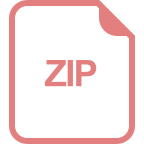
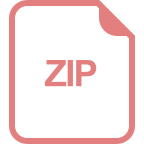
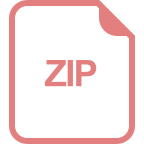
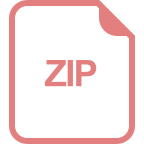
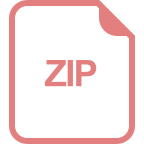
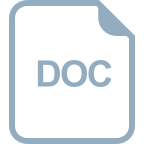


