C#中.NET Framework4.5 封装https GET 带Header参数调用同步返回内容
时间: 2024-09-27 21:06:43 浏览: 6
在C#中,如果你想要使用.NET Framework 4.5通过HTTP GET请求并带有头部参数,同时获取同步返回的内容,可以使用System.Net.Http命名空间下的HttpClient类。以下是简单的示例:
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
class Program
{
static async Task Main()
{
// 创建HttpClient实例
HttpClient client = new HttpClient();
// 设置GET请求的URL和头信息
string url = "http://example.com/api";
Dictionary<string, string> headers = new Dictionary<string, string>
{
{ "Content-Type", "application/json" },
{ "Authorization", "Bearer your_token" } // 这里替换为实际的令牌
};
// 使用HttpMethod.Get方法创建请求,并附带头信息
HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Get, url);
foreach (KeyValuePair<string, string> header in headers)
{
request.Headers.Add(header.Key, header.Value);
}
// 发送异步请求
HttpResponseMessage response = await client.SendAsync(request);
// 检查响应状态码
if (response.IsSuccessStatusCode)
{
// 获取同步返回的内容,假设是JSON格式
string content = await response.Content.ReadAsStringAsync();
Console.WriteLine("Response Content:");
Console.WriteLine(content);
}
else
{
Console.WriteLine($"Request failed with status code: {response.StatusCode}");
}
}
}
```
在这个例子中,`SendAsync`方法用于发送异步请求,然后使用`ReadAsStringAsync`将响应内容读取成字符串。注意,由于.NET Framework 4.5支持的是异步编程,因此这里使用了async和await关键字。
相关推荐
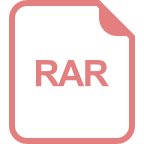
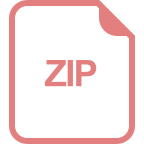
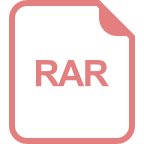














