Python如何调thrift
时间: 2024-09-11 19:07:42 浏览: 15
Thrift是一个接口描述语言和二进制通信协议,它用于定义和创建跨语言的服务。在Python中调用通过Thrift定义的服务,需要以下几个步骤:
1. 使用Thrift IDL定义服务:首先,你需要使用Thrift的接口定义语言(IDL)编写一个接口文件(通常以`.thrift`为扩展名),在这个文件中定义服务的接口和数据类型。
2. 生成代码:使用Thrift编译器根据`.thrift`文件生成指定语言的代码框架。对于Python,Thrift会生成一系列Python模块,包括客户端和服务端的桩代码。
3. 实现服务端:在服务端,你需要根据生成的代码框架来实现定义好的接口。这通常涉及到编写业务逻辑代码,并且使用Thrift提供的服务器框架来启动服务。
4. 编写客户端:在客户端,你需要创建一个Thrift客户端,连接到服务端,然后调用服务端实现的方法。这个过程中,客户端会使用生成的代码来序列化调用参数,发送请求到服务端,并处理服务端返回的结果。
5. 使用Python thrift库:在Python项目中,你需要安装Thrift库,然后使用它来加载生成的模块,并创建客户端实例进行方法调用。
下面是一个简化的例子:
假设有一个名为`HelloService.thrift`的Thrift文件,定义了一个简单的`hello`方法:
```thrift
service HelloService {
string hello(1:string name)
}
```
生成Python代码并实现服务端的步骤:
```python
from thrift.transport import TSocket, TTransport
from thrift.protocol import TBinaryProtocol
from generatedFiles import HelloService
class HelloHandler:
def hello(self, name):
return 'Hello, ' + name
transport = TSocket.TServerSocket(port=9090)
processor = HelloService.Processor(HelloHandler())
server = TThreadPoolServer.TThreadPoolServer(processor, transport)
server.serve()
```
客户端调用服务的步骤:
```python
from thrift.transport import TSocket, TTransport
from thrift.protocol import TBinaryProtocol
from generatedFiles import HelloService
transport = TSocket.TSocket('localhost', 9090)
transport = TTransport.TBufferedTransport(transport)
protocol = TBinaryProtocol.TBinaryProtocol(transport)
client = HelloService.Client(protocol)
transport.open()
greeting = client.hello('World')
print(greeting)
transport.close()
```
相关推荐
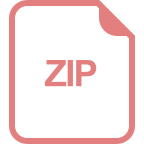
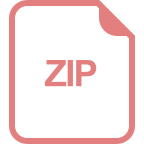
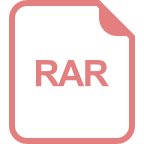














