JSONObject转xml
时间: 2023-09-30 21:09:36 浏览: 103
将JSONObject转换为XML可以使用以下步骤:
1. 创建一个空的XML Document对象。
2. 遍历JSONObject的键值对。
3. 根据每个键值对创建一个XML元素。
4. 如果值是JSONObject或JSONArray类型,则递归调用步骤2-4,否则将值作为文本添加到XML元素中。
5. 将XML元素添加到XML Document中。
6. 将XML Document转换为字符串表示形式。
以下是一个示例代码,演示了如何以Java为例将JSONObject转换为XML:
```java
import org.json.JSONObject;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.transform.OutputKeys;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
public class JSONObjectToXML {
public static void main(String[] args) {
JSONObject jsonObject = new JSONObject();
jsonObject.put("name", "John");
jsonObject.put("age", 30);
jsonObject.put("city", "New York");
try {
Document document = DocumentBuilderFactory.newInstance().newDocumentBuilder().newDocument();
Element rootElement = document.createElement("data");
document.appendChild(rootElement);
convertJSONObjectToXML(jsonObject, rootElement, document);
// 将Document转换为字符串
Transformer transformer = TransformerFactory.newInstance().newTransformer();
transformer.setOutputProperty(OutputKeys.INDENT, "yes");
DOMSource source = new DOMSource(document);
StreamResult result = new StreamResult(System.out);
transformer.transform(source, result);
} catch (ParserConfigurationException | TransformerException e) {
e.printStackTrace();
}
}
private static void convertJSONObjectToXML(JSONObject jsonObject, Element parentElement, Document document) {
for (String key : jsonObject.keySet()) {
Object value = jsonObject.get(key);
Element element = document.createElement(key);
if (value instanceof JSONObject || value instanceof JSONArray) {
convertJSONObjectToXML((JSONObject) value, element, document);
} else {
element.appendChild(document.createTextNode(value.toString()));
}
parentElement.appendChild(element);
}
}
}
```
以上代码将会输出类似以下的XML结果:
```xml
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<data>
<name>John</name>
<age>30</age>
<city>New York</city>
</data>
```
你可以根据自己的需求,进行修改和扩展。
阅读全文
相关推荐


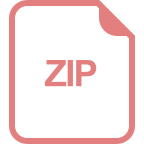
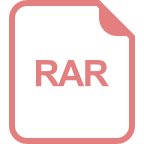
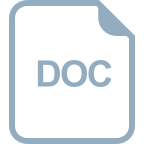










