input = torch.tensor([1, 2, 2, 3, 3, 3, 4, 4, 4, 4,4])找出input张量中出现次数最多的值
时间: 2023-08-19 12:59:08 浏览: 51
你可以使用torch中的torch.bincount()和torch.argmax()函数来找出input张量中出现次数最多的值。下面是一个示例代码:
```python
import torch
# 示例的input张量
input = torch.tensor([1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 4])
# 使用torch.bincount()函数计算每个值出现的次数
counts = torch.bincount(input)
# 使用torch.argmax()函数找出出现次数最多的值的索引
most_common_index = torch.argmax(counts)
# 获取出现次数最多的值
most_common_value = most_common_index.item()
# 打印结果
print("input张量中出现次数最多的值是:", most_common_value)
```
运行以上代码,输出结果将会是:
```
input张量中出现次数最多的值是: 4
```
这样你就成功找出了input张量中出现次数最多的值。
相关问题
帮我修改以下错误 input = torch.tensor(1,2,3,4,5,6) TypeError: tensor() takes 1 positional argument but 6 were given
当使用 `torch.tensor()` 函数时,应该将多个数值参数作为一个列表传递进去,而不是将它们分别作为参数传递。所以,你应该这样写:
```
input = torch.tensor([1, 2, 3, 4, 5, 6])
```
这将创建一个包含给定数值的一维张量。如果你需要一个多维张量,可以将列表嵌套起来,例如:
```
input = torch.tensor([[1, 2], [3, 4], [5, 6]])
```
这将创建一个形状为 `(3, 2)` 的二维张量,包含给定的数值。
随机生成多条类似的数据 : input_question = torch.tensor([[1, 2, 3], [2, 2, 3], [3, 3, 3], [4, 5, 6]], dtype=torch.long, device=torch.device('cpu')) # 输入的问题
如果你想随机生成多条类似的数据,你可以使用PyTorch的随机数生成函数来生成随机的输入问题。下面是一个示例代码,用于生成多条类似的输入问题数据:
```python
import torch
num_samples = 100 # 生成的样本数量
seq_length = 3 # 每个样本的序列长度
input_question = torch.randint(low=1, high=7, size=(num_samples, seq_length), dtype=torch.long)
print(input_question)
```
在上述示例中,我们使用`torch.randint`函数生成了一个形状为`(num_samples, seq_length)`的随机整数张量,范围从1到6。这将生成100条类似的输入问题数据,每个问题由3个整数组成。
你可以根据需要调整`num_samples`和`seq_length`的值来生成所需数量和长度的数据。
希望这个示例能够帮到你!如果还有其他问题,请随时提问。
相关推荐
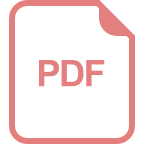
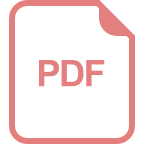
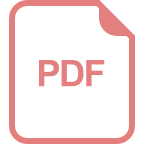












