如何编写一个程序来生成和打印购物小票的功能?
时间: 2024-09-24 10:28:40 浏览: 50
创建一个简单的购物小票程序通常需要以下几个步骤:
1. **数据结构设计**:首先,你需要定义一个数据结构来存储商品信息,比如商品名称、价格和数量。这可以是一个列表或字典,每个元素代表一条商品记录。
```python
class Product:
def __init__(self, name, price, quantity):
self.name = name
self.price = price
self.quantity = quantity
products = []
```
2. **用户输入**:通过循环接收用户的商品添加请求,直到他们完成购物或选择退出。可以使用函数或命令行交互来获取输入。
```python
def add_product():
name = input("请输入商品名称:")
price = float(input("请输入商品价格:"))
quantity = int(input("请输入购买数量:"))
products.append(Product(name, price, quantity))
while True:
add_product()
```
3. **计算总金额**:遍历产品列表,计算总价。
```python
total_amount = 0
for product in products:
total_amount += product.price * product.quantity
print(f"购物小票:")
```
4. **打印购物清单**:显示每种商品的信息以及对应的总价,最后打印出总金额。
```python
for i, product in enumerate(products):
print(f"{i+1}. {product.name} x{product.quantity}: {product.price * product.quantity}")
print(f"\n总金额: {total_amount}")
```
5. **结束程序**:可以选择添加一个结束标志,让用户确认是否结账或继续购物。
```python
confirmation = input("是否还需要继续购物?(y/n): ")
if confirmation.lower() != 'y':
break
```
相关推荐
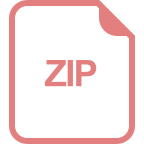
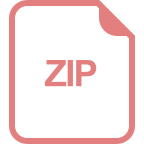
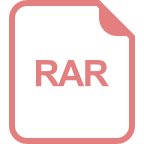














