python normalize np
时间: 2024-04-05 21:22:22 浏览: 16
在Python中,您可以使用NumPy库来对数组进行归一化处理。归一化是将数值缩放到特定范围内的过程,常用的方法是使用最小-最大缩放。
以下是使用NumPy进行归一化的示例代码:
```python
import numpy as np
# 创建一个示例数组
arr = np.array([1, 2, 3, 4, 5])
# 使用最小-最大缩放方法进行归一化
normalized_arr = (arr - np.min(arr)) / (np.max(arr) - np.min(arr))
# 打印归一化后的数组
print(normalized_arr)
```
这将输出归一化后的数组,即将原始数组中的每个元素都缩放到0到1之间。
请注意,这只是归一化的一种方法。根据您的需求,您可能需要使用其他方法来进行归一化。
相关问题
normalize python
Normalizing in Python refers to the process of scaling the values of a variable to a standard range. This is done to make sure that all variables are on the same scale and to improve the performance of machine learning algorithms.
There are different methods for normalizing data in Python, such as:
1. Min-Max Scaling: This method scales the values to a range between 0 and 1. The formula for min-max scaling is:
normalized_value = (value - min_value) / (max_value - min_value)
2. Z-Score Normalization: This method scales the values to have a mean of 0 and a standard deviation of 1. The formula for z-score normalization is:
normalized_value = (value - mean) / standard_deviation
3. Decimal Scaling: This method scales the values by multiplying them by a power of 10. The power of 10 is determined by the maximum absolute value of the variable. The formula for decimal scaling is:
normalized_value = value / 10^k
where k is the number of digits required to represent the maximum absolute value of the variable.
Here is an example of how to normalize data using the Min-Max Scaling method in Python:
```python
from sklearn.preprocessing import MinMaxScaler
import numpy as np
# create sample data
data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# create scaler object
scaler = MinMaxScaler()
# fit and transform data
normalized_data = scaler.fit_transform(data)
print(normalized_data)
```
Output:
```
[[0. 0. 0. ]
[0.5 0.5 0.5]
[1. 1. 1. ]]
```
In this example, the MinMaxScaler object is used to normalize the data. The fit_transform method is called on the scaler object to both fit the scaler to the data and transform the data. The resulting normalized data is printed to the console.
python重写cv2.normalize
可以通过自定义函数来重写cv2.normalize函数。下面是一个简单的例子:
```python
import cv2
import numpy as np
def my_normalize(src, alpha=0, beta=255, norm_type=cv2.NORM_MINMAX):
if norm_type == cv2.NORM_MINMAX:
dst = cv2.normalize(src, None, alpha, beta, cv2.NORM_MINMAX)
elif norm_type == cv2.NORM_INF:
dst = cv2.normalize(src, None, alpha, beta, cv2.NORM_INF)
else:
raise NotImplementedError("Unsupported normalization type: {}".format(norm_type))
return dst
# 测试
img = np.random.randint(0, 256, (3, 3)).astype(np.uint8)
print("Original:\n", img)
print("cv2.normalize:\n", cv2.normalize(img, None, alpha=0, beta=255, norm_type=cv2.NORM_MINMAX))
print("my_normalize:\n", my_normalize(img, alpha=0, beta=255, norm_type=cv2.NORM_MINMAX))
```
这里我们定义了一个my_normalize函数,它接收src(输入数组)、alpha(输出范围的最小值)、beta(输出范围的最大值)、norm_type(归一化类型)四个参数。在函数内部,我们根据不同的归一化类型调用cv2.normalize函数进行处理,最后返回处理结果。我们可以通过调用my_normalize函数来代替cv2.normalize函数。
相关推荐
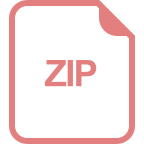
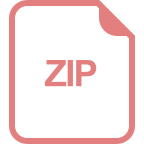












