ifstream文件大小
时间: 2023-12-22 10:18:35 浏览: 126
要获取使用ifstream读取的文件大小,可以通过以下几种方法:
1. 使用C函数:可以使用C函数来获取文件的大小。可以通过使用Windows的_stat函数或者使用C++的ifstream和string库来实现。
2. 使用自定义函数:可以编写一个自定义的函数来获取文件的大小。例如,可以使用get_file_size函数,该函数使用ifstream来读取文件并计算文件的大小。
3. 使用tellg函数:如果你已经打开了文件,并且使用了ifstream对象,你可以使用tellg函数来获取文件的大小。对于已经打开的文件,通过调用tellg()函数可以获取当前文件指针的位置,即文件的大小。例如,可以使用如下代码来获取ifstream文件的大小:
```cpp
ifstream file("filename");
file.seekg(0, file.end);
int size = file.tellg();
```
以上是几种获取ifstream文件大小的方法,选择其中一种方法即可根据需要获取文件的大小。请注意,在使用ifstream读取文件时,如果遇到读取大文件时给出错误的文件大小或无法读取整个文件的问题,可能是由于某些限制或错误的操作导致的。<span class="em">1</span><span class="em">2</span><span class="em">3</span><span class="em">4</span>
相关问题
ifstream 获取文件大小
可以通过使用 `seekg` 和 `tellg` 函数来获取文件大小。以下是一个示例代码:
```c++
#include <fstream>
int getFileSize(std::ifstream& file)
{
int fileSize = 0;
file.seekg(0, std::ios::end); // 将文件指针移到文件末尾
fileSize = file.tellg(); // 获取文件指针当前位置,即文件大小
file.seekg(0, std::ios::beg); // 将文件指针移到文件开头
return fileSize;
}
```
在这个函数中,`seekg` 函数用于将文件指针移到文件的末尾,`tellg` 函数获取文件指针的当前位置,即文件大小。然后,`seekg` 函数再次将文件指针移到文件开头,以便读取文件内容。
在使用该函数时,需要将文件对象作为参数传递给它,例如:
```c++
std::ifstream file("example.txt");
int fileSize = getFileSize(file);
```
C++使用ifstream.read()读二进制文件,并返回读取的buf的大小
使用`ifstream.read()`读取二进制文件可以按照以下步骤进行:
1. 打开二进制文件并创建`ifstream`对象:
```c++
ifstream file("binary_file.bin", ios::in | ios::binary);
```
2. 检查文件是否打开成功:
```c++
if (!file.is_open()) {
cout << "Failed to open file!" << endl;
return -1;
}
```
3. 定义一个缓冲区并分配内存:
```c++
const int BUF_SIZE = 1024;
char* buf = new char[BUF_SIZE];
```
4. 调用`read()`方法读取二进制文件:
```c++
file.read(buf, BUF_SIZE);
```
5. 检查`read()`方法是否成功读取文件:
```c++
if (!file.good()) {
cout << "Failed to read file!" << endl;
return -1;
}
```
6. 获取读取的`buf`的大小:
```c++
int size = file.gcount();
```
7. 关闭文件并释放内存:
```c++
file.close();
delete[] buf;
```
完整代码示例:
```c++
#include <fstream>
#include <iostream>
using namespace std;
int main() {
ifstream file("binary_file.bin", ios::in | ios::binary);
if (!file.is_open()) {
cout << "Failed to open file!" << endl;
return -1;
}
const int BUF_SIZE = 1024;
char* buf = new char[BUF_SIZE];
file.read(buf, BUF_SIZE);
if (!file.good()) {
cout << "Failed to read file!" << endl;
return -1;
}
int size = file.gcount();
cout << "Read " << size << " bytes." << endl;
file.close();
delete[] buf;
return 0;
}
```
阅读全文
相关推荐
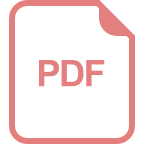
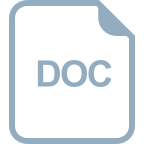
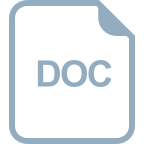
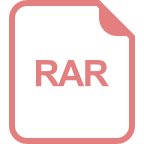
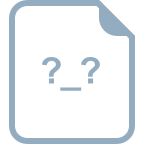
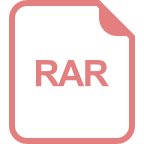
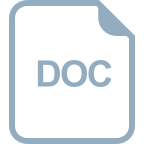









