编写算法,实现串的基本操作Replace(&S,T,V),将串S中所有子串T替换为V,并返回置换次数。
时间: 2024-10-17 11:04:03 浏览: 71
编写一个简单的字符串替换算法,可以按以下步骤实现:
1. 初始化一个变量`count`用于计数替换次数,设置为0。
2. 使用循环遍历整个输入字符串`S`,通过`i`作为当前查找的起始位置。
3. 每次循环内部,创建一个长度等于子串`T`的临时子串`temp`,从索引`i`开始到`i + len(T) - 1`结束。
4. 判断`temp`是否与`T`相等(即它们的内容完全一样),如果相等,则说明找到了需要替换的部分。
5. 如果找到了匹配的子串,将`S`中的这个部分替换为`V`,并在`count`加一。然后移动`i`到`i + len(V)`,以便继续寻找下一个可能的匹配点。
6. 循环结束后,返回`count`作为替换的总次数。
以下是该算法的一个基本伪代码实现:
```python
function Replace(S, T, V):
count = 0
i = 0
while i < length(S):
temp = S[i:i+len(T)]
if temp == T:
S = S[:i] + V + S[i+len(T):]
count += 1
i += len(V)
else:
i += 1
return count
```
相关问题
编写算法,实现串的基本操作Replace(&S,T,V),将串S中所有子串T替换为V,并返回置换次数,C语言
在C语言中,你可以编写一个函数来实现字符串S中的子串T的替换操作,并返回替换的次数。以下是一个简单的实现思路:
```c
#include <stdio.h>
#include <string.h>
// 函数声明
int replace(char *str, const char *search, const char *replace);
// 主函数
int main() {
char S[100], T[10], V[10];
printf("Enter the string S: ");
fgets(S, sizeof(S), stdin); // 防止换行符
S[strlen(S) - 1] = '\0'; // 去除fgets添加的换行符
printf("Enter the substring to be replaced (T): ");
fgets(T, sizeof(T), stdin);
T[strlen(T) - 1] = '\0';
printf("Enter the replacement string (V): ");
fgets(V, sizeof(V), stdin);
V[strlen(V) - 1] = '\0';
int count = replace(S, T, V);
printf("替换次数: %d\n", count);
return 0;
}
// 替换函数
int replace(char *str, const char *search, const char *replace) {
int count = 0, i, j;
for (i = 0; str[i]; i++) {
if (strstr(str + i, search)) { // 搜索子串在原字符串中的位置
j = i;
while (strstr(str + j, search)) { // 找到所有匹配的位置并替换
memmove(str + j + strlen(replace), str + j + strlen(search), strlen(str) - (j + strlen(search)));
memcpy(str + j, replace, strlen(replace));
count++;
j += strlen(replace);
}
}
}
return count;
}
```
这个程序首先从用户那里获取输入的原始字符串S、待替换的子串T和替换后的字符串V。`replace`函数通过`strstr`函数查找子串T在原字符串中的首次出现,然后使用`memmove`和`memcpy`函数进行替换。整个过程会遍历字符串并计算替换次数。
3.编写算法实现串的置换操作Replace(S,T,R), 即将串S中所有与串T相等的子串置换为串R; 或者Replace( S, T, R,&str)。使用递归,C语言
在C语言中,我们可以使用递归来实现字符串的置换操作Replace。这个过程涉及到两个主要步骤:查找子串T在主串S中的所有匹配位置,然后对每个匹配的位置进行替换。以下是一个简单的递归函数示例:
```c
#include <stdio.h>
#include <string.h>
void replace(char *str, const char *pattern, const char *replacement, int pos = 0) {
// 如果pattern结束或者找到了一个匹配,进行替换
if (!*pattern || str[pos] == *pattern) {
if (pos + strlen(pattern) <= strlen(str)) {
memmove(&str[pos + strlen(replacement)], &str[pos], strlen(&str[pos]) - pos);
memcpy(str + pos, replacement, strlen(replacement));
printf("Replaced \"%s\" at position %d with \"%s\"\n", pattern, pos, replacement);
}
// 继续寻找下一个匹配
return replace(str, pattern + 1, replacement, pos + 1);
} else {
return replace(str, pattern, replacement, pos + 1); // 没有找到匹配,移动到下一个字符继续搜索
}
}
int main() {
char str[] = "Hello, world! This is a test.";
const char *T = "is";
const char *R = "was";
replace(str, T, R);
printf("%s\n", str);
return 0;
}
```
在这个例子中,`replace` 函数接收三个参数:原始字符串 `str`,要查找的子串 `pattern` 和替换成的新字符串 `replacement`。函数首先检查当前字符是否与 `pattern` 的第一个字符匹配,如果匹配,它会比较子串长度来确认是否真的是一次完整的匹配,然后进行替换并更新指针。如果不匹配,则继续递归在下一个字符上搜索。
注意,这个实现假设输入字符串都是有效的,并且 `replacement` 的长度不会超过替换后的总字符串长度。在实际应用中,需要添加错误处理和边界条件检查。
阅读全文
相关推荐
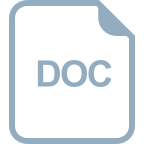
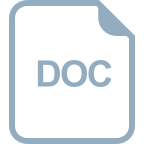
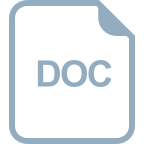













