写一段wpf代码最常用的单元测试代码
时间: 2024-09-14 08:02:51 浏览: 92
在WPF应用程序中进行单元测试,我们通常会使用一些专门的测试框架来帮助我们模拟WPF应用程序中的依赖项和用户界面元素。一个常用的单元测试库是Microsoft的xUnit,它可以用来测试逻辑代码。但若要测试WPF特定的代码,如与依赖项属性或命令绑定等相关的功能,我们可能需要使用Moq来模拟这些依赖项,以及使用FluentAssertions来验证复杂的对象图。
下面是一个使用xUnit、Moq和FluentAssertions编写的简单示例代码,用于测试WPF中的一个ViewModel。
```csharp
using Xunit;
using Moq;
using FluentAssertions;
using System.Windows.Input;
using MyWpfApp.ViewModels;
namespace MyWpfApp.Tests
{
public class MainViewModelTests
{
[Fact]
public void Command_ShouldExecute()
{
// Arrange
var mockService = new Mock<IService>();
var viewModel = new MainViewModel(mockService.Object);
// Act
viewModel.DoSomethingCommand.Execute(null);
// Assert
mockService.Verify(x => x.DoSomething(), Times.Once);
viewModel.StatusMessage.Should().Be("Done!");
}
}
public interface IService
{
void DoSomething();
}
public class MainViewModel
{
private readonly IService _service;
public ICommand DoSomethingCommand { get; }
public string StatusMessage { get; private set; }
public MainViewModel(IService service)
{
_service = service;
DoSomethingCommand = new RelayCommand(DoSomething);
}
private void DoSomething()
{
_service.DoSomething();
StatusMessage = "Done!";
}
}
public class RelayCommand : ICommand
{
private readonly Action _execute;
public event EventHandler CanExecuteChanged;
public RelayCommand(Action execute)
{
_execute = execute;
}
public bool CanExecute(object parameter)
{
return true; // For simplicity, always return true
}
public void Execute(object parameter)
{
_execute?.Invoke();
}
}
}
```
在这个测试中,我们创建了一个模拟的服务(`IService`),并验证当执行一个命令(`DoSomethingCommand`)时,是否调用了服务的方法,并且ViewModel的状态消息是否正确更新。
阅读全文
相关推荐
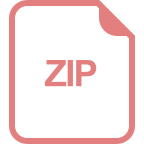
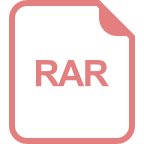
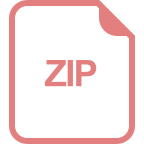
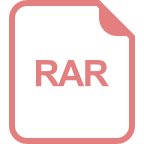
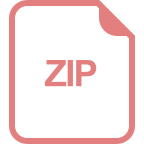
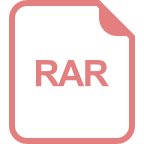
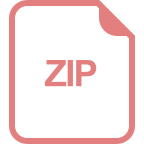
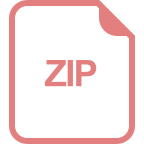
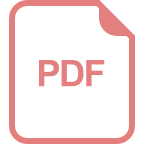
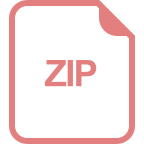
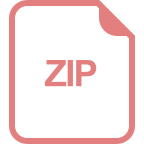
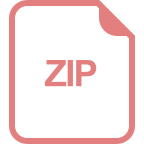
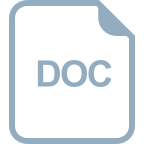
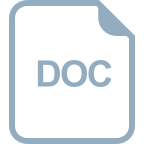
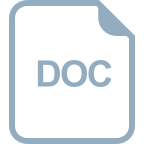
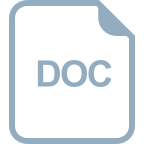
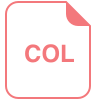
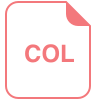
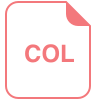