javaswing文本窗口
时间: 2025-01-01 08:31:41 浏览: 20
### Java Swing 中实现文本窗口
在Java Swing中,`JTextArea` 和 `JEditorPane` 是两个常用的组件来显示和编辑多行文本。下面分别介绍这两种方式并提供相应的代码示例。
#### 使用 JTextArea 显示和编辑文本
`JTextArea` 提供了一个可滚动的区域用于展示纯文本内容。可以通过设置自动滚动属性使文本框能够随着输入的内容增长而调整视图位置[^2]。
```java
import javax.swing.*;
import java.awt.*;
public class TextAreaExample {
public static void main(String[] args) {
// 创建主框架
JFrame frame = new JFrame("Text Area Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 设置布局管理器
Container container = frame.getContentPane();
container.setLayout(new BorderLayout());
// 初始化 JTextArea 组件
JTextArea textArea = new JTextArea(10, 40);
// 启用自动滚动功能
textArea.setLineWrap(true); // 开启换行模式
textArea.setWrapStyleWord(true); // 按单词而不是字符断开
// 将 JTextArea 放入 JScrollPane 中以便于当内容超出可视范围时能正常滚动查看
JScrollPane scrollPane = new JScrollPane(textArea);
container.add(scrollPane, BorderLayout.CENTER);
// 展示窗口
frame.pack();
frame.setVisible(true);
}
}
```
#### 使用 JEditorPane 加载 HTML 文档或其他富文本格式
对于更复杂的文本需求,比如支持HTML标签或者其他标记语言编写的文档,则可以选择使用 `JEditorPane` 来加载这些类型的文件。它允许直接解析特定MIME类型的数据源,并将其渲染成对应的视觉效果[^1]。
```java
import javax.swing.*;
import java.io.IOException;
import java.net.URL;
public class EditorPaneExample {
public static void main(String[] args) throws IOException {
// 创建主框架
JFrame frame = new JFrame("Editor Pane Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 获取资源路径下的html文件URL对象
URL url = ClassLoader.getSystemResource("example.html");
// 初始化 JEditorPane 并指定要读取的 MIME 类型 (这里是 "text/html")
JEditorPane editorPane = new JEditorPane(url.toExternalForm());
editorPane.setEditable(false); // 不允许修改已加载页面
// 添加到容器内
Container container = frame.getContentPane();
container.add(new JScrollPane(editorPane), BorderLayout.CENTER);
// 展示窗口
frame.setSize(800, 600);
frame.setVisible(true);
}
}
```
这两个例子展示了如何利用 `JTextArea` 处理简单文本以及通过 `JEditorPane` 解析复杂结构化文本的方式,在实际开发过程中可以根据具体应用场景选择合适的技术方案。
阅读全文
相关推荐






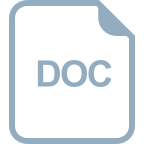
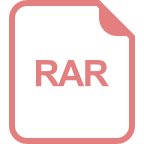
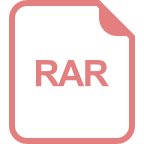
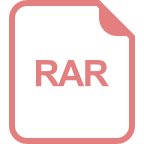









