fsql.Update<point>().SetSource(pList).IgnoreColumns(a => new { a.occupy, a.scene, a.area }).ExecuteAffrows()必须要指定id吗
时间: 2024-12-17 15:34:17 浏览: 4
在`fsql.Update<User>()`示例中[^1],Update操作通常会通过条件查询更新特定记录,而`Where(u => u.Id == user1.Id)`这部分明确指定了要更新的Id。对于`fsql.Update<point>()`的情况,如果想要基于某些属性而不是ID来更新点对象(Point),则可能需要明确指定更新条件,因为`Update`操作默认会依据主键(如ID)进行更新。
然而,如果你想要忽略`a.occupy`, `a.scene`, `a.area`这些列而不必指定它们的ID,你可以这样做:
```csharp
fsql.Update<point>()
.SetSource(pList)
.IgnoreColumns(x => x.occupy, x => x.scene, x => x.area)
.WithoutCondition() // 如果不需要其他标准的更新条件,可以添加这行来避免自动使用主键
.ExecuteAffrows();
```
但请注意,不指定更新条件可能会导致所有匹配的记录都被更新,因此在实际应用中,最好还是提供一个明确的`Where`子句来限制更新哪些记录。
相关问题
FreeSql中,fsql.Update<point>().SetSource(pList).IgnoreColumns(a => new { a.scene, a.area }).ExecuteAffrows();语句添加当scene=2时,忽略列scene
在FreeSql中,`fsql.Update<User>()` 方法用于更新数据,`SetSource()` 是设置要更新的数据源,而 `Where()` 是指定更新的条件。`IgnoreColumns()` 方法允许你在执行更新操作时忽略某些列。在这个示例中,`fsql.Update<point>()` 应该替换为具体的实体类名(如 `Update<Point>()`),`pList` 是你想更新的对象集合。
如果你想在场景 (`scene`) 值等于 2 的时候忽略 `scene` 列,你可以这样编写代码:
```csharp
fsql.Update<Point>()
.SetSource(pList)
.When(u => u.scene == 2, ignoredColumns => ignoredColumns.Add("scene")) // 使用When方法来指定场景为2时忽略scene列
.Where(u => u.Id == someId) // 根据ID查找需要更新的点
.ExecuteAffrows();
```
这里假设`someId`是你想匹配的具体ID。这段代码会在满足`u.scene == 2`这个条件时,更新操作不会更改`scene`字段的值。
fsql.Update<User>()语句批量更新时,不按照主键更新,按照另外的字段更新
在Freesql (十四) 中,批量更新数据时,通常会考虑数量参数量和每批分割的数据大小来提高性能。如果想要按照除主键之外的字段进行批量更新而不按主键顺序,你可以直接在`Update<User>()`语句中指定这些字段及其新值,例如:
```csharp
using(FreeSql fsql = new FreeSql())
{
// 假设User表有一个名为'SpecialField'的字段用于更新
var updateConditions = "[SpecialField] = @value"; // 更新条件
var updateValues = new { value = "your_new_value" }; // 新的字段值
fsql.Update<User>()
.Set(updateConditions)
.Where($"[SomeOtherField] > @batchStart", new { batchStart = "batch_start_value"})
.BatchSize(batchSize: yourBatchSize) // 按照特定大小分割批次
.Execute(); // 执行批量更新
}
```
这里,`$batchStart`是你想要开始更新的特定字段值,`yourBatchSize`应该与上述的数据库分割规则相匹配。注意,实际操作中可能需要根据具体的数据库连接配置调整`BatchSize`。
阅读全文
相关推荐
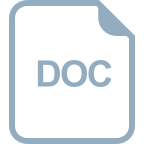
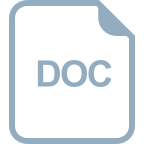
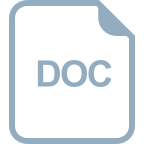




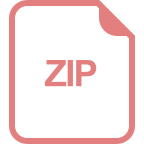
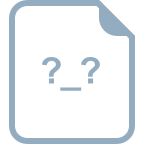
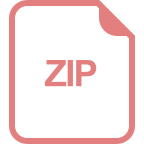
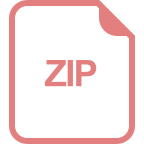
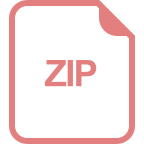
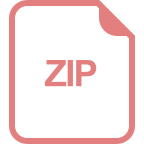
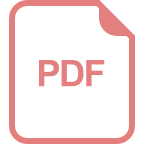


