1.重新搭建一个web项目: ⒉.利用dao+service层的方式实现从dept表中删除部门数据,前提是该部门下面没有员工 3.拓展:将news项目当中的删除和新增改成service层的方式 代码
时间: 2024-04-12 13:32:18 浏览: 19
根据你的需求,我可以给你提供一个示例代码,演示如何利用dao+service层的方式实现从dept表中删除部门数据,前提是该部门下面没有员工。请注意,以下示例代码是基于Java语言和Spring框架的。
首先,创建Dept实体类,用于映射dept表结构:
```java
public class Dept {
private int id;
private String name;
// 其他属性和方法省略
}
```
接下来,创建DeptDAO接口和实现类,用于封装对dept表的数据库操作:
```java
public interface DeptDAO {
void deleteDept(int deptId);
}
@Repository
public class DeptDAOImpl implements DeptDAO {
@Autowired
private JdbcTemplate jdbcTemplate;
@Override
public void deleteDept(int deptId) {
String sql = "DELETE FROM dept WHERE id = ?";
jdbcTemplate.update(sql, deptId);
}
}
```
然后,创建DeptService接口和实现类,用于处理业务逻辑:
```java
public interface DeptService {
void deleteDept(int deptId) throws Exception;
}
@Service
public class DeptServiceImpl implements DeptService {
@Autowired
private DeptDAO deptDAO;
@Override
public void deleteDept(int deptId) throws Exception {
// 先检查该部门是否有员工
if (hasEmployees(deptId)) {
throw new Exception("该部门下存在员工,无法删除");
}
deptDAO.deleteDept(deptId);
}
private boolean hasEmployees(int deptId) {
// TODO: 检查该部门是否有员工的逻辑
return false;
}
}
```
最后,在控制器中调用DeptService的方法来处理删除部门的请求:
```java
@RestController
@RequestMapping("/dept")
public class DeptController {
@Autowired
private DeptService deptService;
@DeleteMapping("/{deptId}")
public ResponseEntity<String> deleteDept(@PathVariable int deptId) {
try {
deptService.deleteDept(deptId);
return ResponseEntity.ok("删除成功");
} catch (Exception e) {
return ResponseEntity.badRequest().body(e.getMessage());
}
}
}
```
这样,当你发送 DELETE 请求到 "/dept/{deptId}" 路径时,控制器会调用DeptService的deleteDept方法来删除部门数据。
对于将news项目中的删除和新增功能改成service层的方式,你可以按照类似的思路进行修改。希望以上示例代码能对你有所帮助!如果还有其他问题,请随时提问。
相关推荐
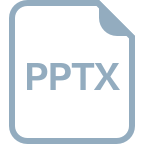
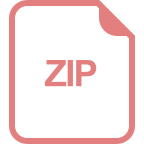
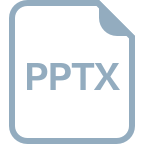














