easyx推箱子参考文献
时间: 2024-07-01 18:00:32 浏览: 200
EasyX是一个轻量级的游戏开发框架,主要用于Windows平台,提供了一些基本的游戏开发工具和库。对于"推箱子"这类游戏的开发,虽然EasyX本身并不直接支持这种复杂策略型游戏的全部功能,但它可以作为基础框架,帮助开发者处理游戏逻辑和用户界面。
如果你想了解EasyX在制作推箱子游戏中的应用,可能没有特定的官方参考文献,因为EasyX更多是提供一个通用的游戏开发环境,而不是专门针对某个游戏设计的。然而,你可以参考以下资源来结合EasyX进行推箱子游戏开发:
- EasyX官方文档:https://easyx.readthedocs.io/,这里有基本的API和教程,可以帮助你理解如何在EasyX中创建游戏对象和处理事件。
- EasyX论坛或社区:如CSDN或GitHub上的EasyX讨论区,那里可能有开发者分享过使用EasyX做游戏开发的经验和技巧。
- 自学编程书籍,特别是关于C++和游戏开发的部分,这些内容通常会介绍如何将游戏逻辑应用到任何图形库上,包括EasyX。
- 利用网络资源,如博客文章、教程视频或Stack Overflow上的问题和答案,寻找关于C++游戏开发和EasyX的具体实施案例。
如果你对EasyX之外的推箱子游戏算法有兴趣,可以查阅经典的计算机科学书籍,比如《人工智能:一种现代方法》(Artificial Intelligence: A Modern Approach)或专门的AI游戏开发教材。
相关问题
easyx推箱子(分文件)
好的,下面是推箱子的分文件实现:
首先,我们需要新建4个文件:
- main.cpp:主函数文件
- game.h:游戏类头文件
- game.cpp:游戏类实现文件
- map.h:地图类头文件
接着,我们来编写代码:
map.h
```cpp
#pragma once
#include <vector>
#include <string>
class Map {
public:
Map();
~Map();
void loadMap(const std::string& mapPath);
int getWidth() const;
int getHeight() const;
int getBoxCount() const;
char getMapElement(int x, int y) const;
bool isCompleted() const;
bool moveBox(int x1, int y1, int x2, int y2);
void printMap() const;
private:
int width, height, boxCount;
std::vector<char> mapData;
std::vector<int> boxPositions;
};
```
game.h
```cpp
#pragma once
#include "map.h"
class Game {
public:
Game();
~Game();
void run();
private:
Map map;
bool isGameOver;
void handleInput();
void update();
void render() const;
};
```
game.cpp
```cpp
#include "game.h"
#include <iostream>
#include <conio.h>
Game::Game() : isGameOver(false) {}
Game::~Game() {}
void Game::run() {
map.loadMap("map.txt");
while (!isGameOver) {
handleInput();
update();
render();
}
}
void Game::handleInput() {
char ch = _getch();
switch (ch) {
case 'w':
case 'W':
map.moveBox(0, -1, 0, -2);
break;
case 's':
case 'S':
map.moveBox(0, 1, 0, 2);
break;
case 'a':
case 'A':
map.moveBox(-1, 0, -2, 0);
break;
case 'd':
case 'D':
map.moveBox(1, 0, 2, 0);
break;
case 'q':
case 'Q':
isGameOver = true;
break;
}
}
void Game::update() {
if (map.isCompleted()) {
std::cout << "Congratulations! You completed the game!\n";
isGameOver = true;
}
}
void Game::render() const {
system("cls");
map.printMap();
}
int main() {
Game game;
game.run();
return 0;
}
```
map.cpp
```cpp
#include "map.h"
#include <fstream>
#include <iostream>
Map::Map() : width(0), height(0), boxCount(0) {}
Map::~Map() {}
void Map::loadMap(const std::string& mapPath) {
std::ifstream mapFile(mapPath);
if (!mapFile.is_open()) {
std::cerr << "Failed to open map file: " << mapPath << std::endl;
return;
}
std::string line;
while (std::getline(mapFile, line)) {
if (line.empty()) {
continue;
}
if (width == 0) {
width = line.size();
}
for (char ch : line) {
switch (ch) {
case '@':
boxPositions.push_back(mapData.size());
// fall through
case '.':
case '#':
mapData.push_back(ch);
break;
default:
std::cerr << "Invalid map element: " << ch << std::endl;
break;
}
}
height++;
}
boxCount = boxPositions.size();
}
int Map::getWidth() const {
return width;
}
int Map::getHeight() const {
return height;
}
int Map::getBoxCount() const {
return boxCount;
}
char Map::getMapElement(int x, int y) const {
return mapData[y * width + x];
}
bool Map::isCompleted() const {
for (int i = 0; i < boxCount; i++) {
if (mapData[boxPositions[i]] != '.') {
return false;
}
}
return true;
}
bool Map::moveBox(int x1, int y1, int x2, int y2) {
int pos1 = (y1 + y2) * width + x1 + x2;
int pos2 = y1 * width + x1;
if (pos1 < 0 || pos1 >= width * height) {
return false;
}
if (mapData[pos1] == '#' || mapData[pos1] == '$') {
return false;
}
if (mapData[pos1] == '.') {
mapData[pos1] = '$';
} else {
mapData[pos1] = '*';
}
if (mapData[pos2] == '$') {
mapData[pos2] = '.';
} else {
mapData[pos2] = '@';
}
for (int i = 0; i < boxCount; i++) {
if (boxPositions[i] == pos2) {
boxPositions[i] = pos1;
break;
}
}
return true;
}
void Map::printMap() const {
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
std::cout << getMapElement(x, y);
}
std::cout << std::endl;
}
}
```
这样,我们就把推箱子游戏的代码分成了4个文件,更加清晰易懂。
阅读全文
相关推荐
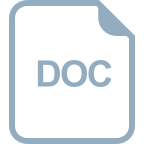
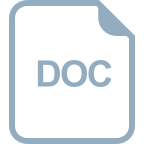
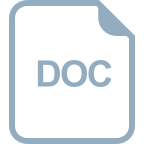
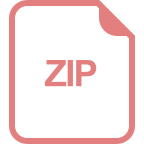
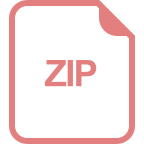
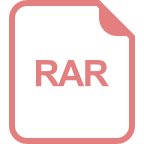
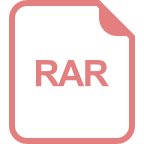
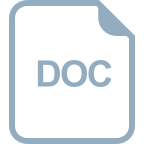
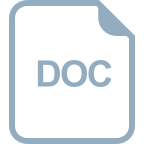
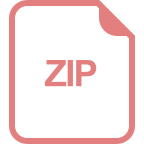
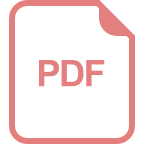
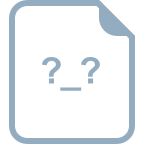
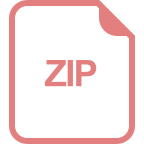
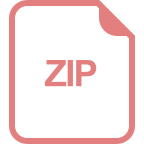
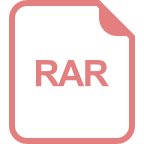
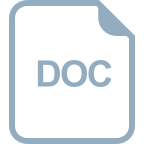