如何使用C语言编写一个程序来计算并显示给定字符串中各个元音字母(A, E, I, O, U)的出现次数?
时间: 2024-11-09 13:21:20 浏览: 45
在C语言中,你可以通过以下几个步骤编写一个程序来计算并显示给定字符串中元音字母的数量:
1. **包含头文件**:开始时,需要包含`stdio.h`用于输入和输出,以及`ctype.h`用于字符分类。
```c
#include <stdio.h>
#include <ctype.h>
```
2. **函数声明**:创建一个名为`count_vowels`的函数,它接受一个字符串作为参数,并返回元音字母的计数。
```c
int count_vowels(const char *str);
```
3. **主函数**:在main()函数中获取用户输入的字符串,调用`count_vowels`函数,然后输出结果。
```c
int main() {
const char *input = "example string";
int vowel_count = count_vowels(input);
printf("The number of vowels in the given string is: %d\n", vowel_count);
return 0;
}
```
4. **`count_vowels` 函数实现**:在这个函数中遍历输入的字符串,检查每个字符是否是元音字母。
```c
int count_vowels(const char *str) {
int count = 0;
for (const char *p = str; *p != '\0'; ++p) {
if (isalpha(*p) && (*p == 'A' || *p == 'E' || *p == 'I' || *p == 'O' || *p == 'U')) {
count++;
}
}
return count;
}
```
这个函数首先判断字符是否为字母(`isalpha()`),如果是并且在元音列表内,就增加计数器。最后返回计数值。
阅读全文
相关推荐
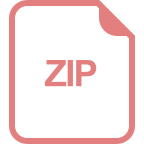
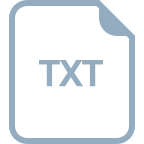
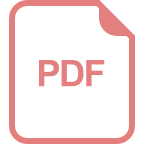

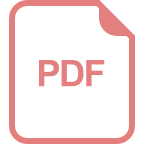
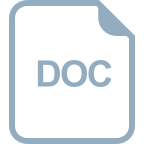
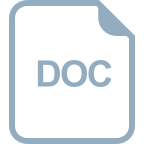
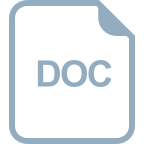
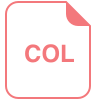








